Sort the student list in the ascending order of student names and print them all on the screen
时间: 2024-03-21 20:39:27 浏览: 30
Sure, here's the updated code to sort the students by name in ascending order and print them to the screen:
```
import java.util.*;
public class Main {
public static void main(String[] args) {
ArrayList<Student> students = new ArrayList<Student>();
// Read students from file
try {
Scanner scanner = new Scanner(new File("students.txt"));
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] parts = line.split(",");
String name = parts[0];
ArrayList<String> hobbies = new ArrayList<String>();
for (int i = 1; i < parts.length; i++) {
hobbies.add(parts[i]);
}
Student student = new Student(name, hobbies);
students.add(student);
}
scanner.close();
} catch (FileNotFoundException e) {
System.out.println("File not found!");
}
// Sort students by name in ascending order
Collections.sort(students, new Comparator<Student>() {
@Override
public int compare(Student s1, Student s2) {
return s1.getName().compareTo(s2.getName());
}
});
// Print sorted list of students
for (Student student : students) {
System.out.println(student.getName() + ": " + student.getHobbies());
}
}
}
```
This code uses the Collections.sort() method to sort the ArrayList of students by name in ascending order. It also uses an anonymous inner class that implements the Comparator interface to define the sorting order. Finally, it loops through the sorted list of students and prints each student's name and hobbies to the console.
相关推荐
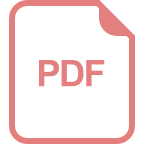
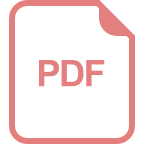
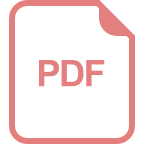














