create a class called Student. Every Student will contain a name (String) and an ArrayList<String> storing hobbies. Then, you will add all those students from the file into an ArrayList<Student>, with each Student having a separate name and ArrayList of hobbies. Here is an example file containing students (the first line will always represent yourself). NOTE: eventually, we will have a different file containing all our real names and hobbies so that we could find out with how many people each of us share the same hobby. Vitaly,table tennis,chess,hacking Sean,cooking,guitar,rainbow six Nolan,gym,piano,reading,video games Jack,cooking,swimming,music Ray,piano,video games,volleyball Emily,crochet,drawing,gardening,tuba,violin Hudson,anime,video games,trumpet Matt,piano,Reading,video games,traveling Alex,swimming,video games,saxophone Roman,piano,dancing,art Teddy,chess,lifting,swimming Sarah,baking,reading,singing,theatre Maya,violin,knitting,reading,billiards Amy,art,gaming,guitar,table tennis Daniel,video games,tennis,soccer,biking,trumpet Derek,cooking,flute,gaming,swimming,table tennis Daisey,video games,guitar,cleaning,drawing,animated shows,reading,shopping Lily,flute,ocarina,video games,baking Stella,roller skating,sudoku,watching baseball,harp Sophie,viola,ukulele,piano,video games Step 2. Sort the student list in the ascending order of student names and print them all on the screen
时间: 2024-03-05 10:06:47 浏览: 91
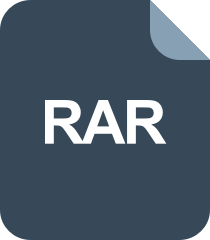
建立一个名为Student的类,该类有以下几个私有成员变量:学生姓名、学号、性别、年龄。还有以下两个成员函数:一个用于初始化学生姓名、学号、性别和年龄的构造函数,一个用于输出学生信息的函数。编写一个主函数。声明一个学学生对象。然后调用成员函数在屏幕输出学生信息。

Sure, here is an example implementation in Java:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
public class Student {
private String name;
private ArrayList<String> hobbies;
public Student(String name, ArrayList<String> hobbies) {
this.name = name;
this.hobbies = hobbies;
}
public String getName() {
return name;
}
public ArrayList<String> getHobbies() {
return hobbies;
}
public static void main(String[] args) {
ArrayList<Student> students = new ArrayList<>();
try (BufferedReader br = new BufferedReader(new FileReader("students.txt"))) {
String line;
while ((line = br.readLine()) != null) {
String[] parts = line.split(",");
String name = parts[0];
ArrayList<String> hobbies = new ArrayList<>();
for (int i = 1; i < parts.length; i++) {
hobbies.add(parts[i]);
}
students.add(new Student(name, hobbies));
}
} catch (IOException e) {
System.err.format("IOException: %s%n", e);
}
Collections.sort(students, (s1, s2) -> s1.getName().compareTo(s2.getName()));
for (Student student : students) {
System.out.println(student.getName() + ": " + student.getHobbies());
}
}
}
```
This code reads the student data from a file called "students.txt" (in the same directory as the Java file), creates a `Student` object for each line, and stores them in an `ArrayList`. It then sorts the list by student name using a lambda expression, and finally prints out each student's name and hobbies using a `for` loop.
阅读全文
相关推荐
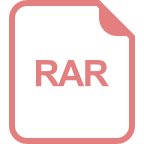
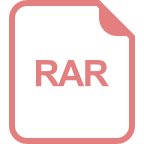



<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <title>个人介绍</title> <style> /* 样式表 */ /* 轮播图区域 */ .slideshow { position: relative; width: 100%; height: 300px; overflow: hidden; } .slideshow img { position: absolute; top: 0; left: 0; width: 100%; height: 100%; object-fit: cover; opacity: 0; transition: opacity 1s ease-in-out; } .slideshow img.active { opacity: 1; } /* 导航栏区域 */ nav { background-color: #333; color: #fff; display: flex; justify-content: space-between; padding: 10px; } nav a { color: #fff; text-decoration: none; margin: 0 10px; } </style></head><body> 首页 关于我 联系我
欢迎来到我的个人网站 我叫XXX,是一名XXX。我喜欢XXX,平时喜欢XXX。欢迎联系我,我的邮箱是XXX。 <script> // 轮播图部分的脚本 var images = document.querySelectorAll('.slideshow img'); var currentImageIndex = 0; var slideshowInterval = setInterval(nextImage, 3000); function nextImage() { images[currentImageIndex].classList.remove('active'); currentImageIndex = (currentImageIndex + 1) % images.length; images[currentImageIndex].classList.add('active'); } </script></body></html>你这个代码图片显示不完全,如何完整显示图片











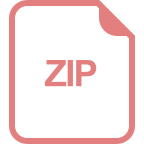
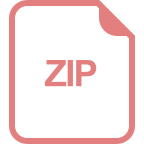