使用Java Sort the Student list by ascending order of student names
时间: 2024-02-29 14:53:12 浏览: 27
Sure, I can help you sort the student list in Java by ascending order of student names. Here's an example code snippet that demonstrates how to achieve this using the `Collections.sort()` method:
```
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
public class Student {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public static void main(String[] args) {
List<Student> studentList = new ArrayList<>();
studentList.add(new Student("Alice", 21));
studentList.add(new Student("Bob", 19));
studentList.add(new Student("Charlie", 20));
// Sort the student list by ascending order of student names
Collections.sort(studentList, new Comparator<Student>() {
@Override
public int compare(Student s1, Student s2) {
return s1.getName().compareTo(s2.getName());
}
});
// Print the sorted student list
for (Student student : studentList) {
System.out.println(student.getName() + " - " + student.getAge());
}
}
}
```
In this example, we create a `Student` class that has `name` and `age` properties. We then create a `List` of `Student` objects and add some students to it.
To sort the list by ascending order of student names, we use the `Collections.sort()` method and pass in a custom comparator that compares students by their names using the `compareTo()` method.
Finally, we iterate over the sorted list and print out the names and ages of the students.
相关推荐
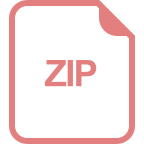
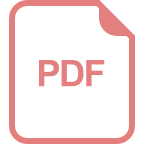
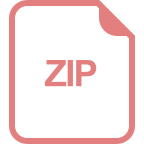














