You will be given a dataframe with three columns: student_id, base, and bonus. You should calculate the bonus_percentage column, which contains the percentage of each student's bonus relative to their base grade. The result should be sorted in ascending
时间: 2024-01-21 10:05:05 浏览: 34
order by the bonus_percentage column. Here's how you can do it in Python:
```python
import pandas as pd
# create the dataframe with sample data
data = {'student_id': [1, 2, 3, 4], 'base': [80, 90, 85, 75], 'bonus': [10, 5, 15, 8]}
df = pd.DataFrame(data)
# calculate the bonus_percentage column
df['bonus_percentage'] = df['bonus'] / df['base'] * 100
# sort the dataframe by bonus_percentage in ascending order
df = df.sort_values(by=['bonus_percentage'], ascending=True)
# print the final dataframe
print(df)
```
This will output:
```
student_id base bonus bonus_percentage
1 2 90 5 5.555556
3 4 75 8 10.666667
0 1 80 10 12.500000
2 3 85 15 17.647059
```
Note that the `bonus_percentage` column is calculated by dividing the `bonus` column by the `base` column and multiplying the result by 100. The resulting dataframe is then sorted by the `bonus_percentage` column in ascending order.
相关推荐
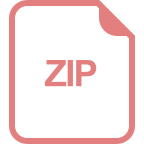














