用C语言拼接char拼接hello world
时间: 2023-09-04 11:12:59 浏览: 85
```c
#include <stdio.h>
#include <string.h>
int main(void) {
char str1[] = "hello";
char str2[] = "world";
char result[strlen(str1) + strlen(str2) + 1]; // +1 for null terminator
strcpy(result, str1); // copy str1 into result
strcat(result, str2); // concatenate str2 onto result
printf("%s\n", result); // print "hello world"
return 0;
}
```
相关问题
C语言用char类型合并Hello和World(不用strcpy)
在C语言中,如果你想要将字符串"Hello"和"World"合并到一个`char`类型的数组中,并且不使用`strcpy`函数,可以使用字符数组拼接的方式。这里是一个简单的示例:
```c
#include <stdio.h>
#define MAX_LENGTH 50 // 假设数组最大长度为50
int main() {
char str1[] = "Hello";
char str2[] = "World";
char result[MAX_LENGTH]; // 结果数组
int i = 0, j = 0, k = 0;
while (str1[i] != '\0') { // 遍历str1
result[k++] = str1[i++]; // 将每个字符添加到结果数组
}
result[k++] = ' '; // 在两个字符串之间添加空格
while (str2[j] != '\0') { // 遍历str2
result[k++] = str2[j++];
}
result[k] = '\0'; // 添加结束符
printf("合并后的字符串: %s\n", result);
return 0;
}
```
这个程序首先遍历`str1`并逐个将其字符复制到`result`数组,然后添加一个空格,再遍历`str2`并将字符添加到数组中,最后在末尾添加一个结束符`\0`。
C语言用char类型将Hello和World合并到一个数组
在C语言中,你可以使用`char`类型的字符数组来存储字符串"Hello"和"World"。这里是一个简单的例子:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[20]; // 定义一个足够大的数组来存放两个字符串,假设每个字符串最多9个字符加上空字符'\0'
// 将两个字符串拼接起来
strncpy(str, "Hello", sizeof(str) - 1); // 首先复制"Hello"
str[strlen("Hello")] = ' '; // 在"Hello"结尾添加空格
strcat(str, "World"); // 然后拼接"World"
// 输出结果
printf("%s\n", str);
return 0;
}
```
注意:`strncpy`函数用于防止溢出,因为它只复制指定长度的内容,并在到达目的地数组边界前自动停止。`strlen`用于计算第一个字符串的长度,包括结束的空字符`\0`。`strcat`用于连接两个字符串。
阅读全文
相关推荐
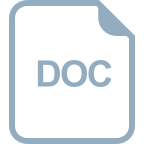
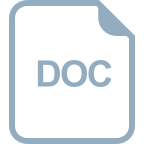
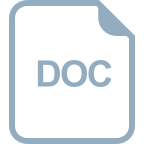



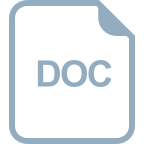









