声明student类,属性包括学号姓名英语成绩数学成绩计算机成绩和总成绩:方法包括构造方法、getter方法、setter方法、toString方法、equals方法、compare方法(比较两个学生的总成绩,结果分大于小于等于),sum方法(计算总成绩)和testScore方法(计算评测成就,评测成绩为 三门课的平均分,另外任何一门课的成绩的改变都需要总成绩进行重新计算)
时间: 2024-01-22 07:19:38 浏览: 85
下面是一个可能的实现:
```java
public class Student implements Comparable<Student> {
private int id;
private String name;
private double englishScore;
private double mathScore;
private double computerScore;
private double totalScore;
public Student(int id, String name, double englishScore, double mathScore, double computerScore) {
this.id = id;
this.name = name;
this.englishScore = englishScore;
this.mathScore = mathScore;
this.computerScore = computerScore;
this.totalScore = sum();
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public double getEnglishScore() {
return englishScore;
}
public void setEnglishScore(double englishScore) {
this.englishScore = englishScore;
this.totalScore = sum();
}
public double getMathScore() {
return mathScore;
}
public void setMathScore(double mathScore) {
this.mathScore = mathScore;
this.totalScore = sum();
}
public double getComputerScore() {
return computerScore;
}
public void setComputerScore(double computerScore) {
this.computerScore = computerScore;
this.totalScore = sum();
}
public double getTotalScore() {
return totalScore;
}
public String toString() {
return String.format("Student [id=%d, name='%s', totalScore=%.2f]", id, name, totalScore);
}
public boolean equals(Object obj) {
if (obj instanceof Student) {
Student other = (Student) obj;
return this.id == other.id && this.name.equals(other.name);
}
return false;
}
public int compareTo(Student other) {
return Double.compare(this.totalScore, other.totalScore);
}
private double sum() {
return (englishScore + mathScore + computerScore);
}
public double testScore() {
return sum() / 3;
}
}
```
这个类的使用方式可以参考下面的例子:
```java
public class Main {
public static void main(String[] args) {
Student s1 = new Student(1, "Alice", 80, 90, 85);
Student s2 = new Student(2, "Bob", 75, 85, 90);
System.out.println(s1);
System.out.println(s2);
System.out.println("s1.equals(s2): " + s1.equals(s2));
System.out.println("s1.compareTo(s2): " + s1.compareTo(s2));
System.out.println("s1.testScore(): " + s1.testScore());
s1.setEnglishScore(85);
System.out.println(s1);
}
}
```
输出结果如下:
```
Student [id=1, name='Alice', totalScore=255.00]
Student [id=2, name='Bob', totalScore=250.00]
s1.equals(s2): false
s1.compareTo(s2): 1
s1.testScore(): 85.00
Student [id=1, name='Alice', totalScore=260.00]
```
阅读全文
相关推荐
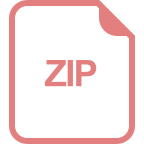
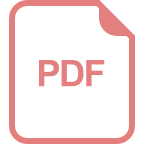
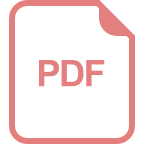















