(1)声明Student类。 属性包括:学号、姓名、英语成绩、数学成绩、计算机成绩和总成绩。 方法包括:构造方法、getter方法、setter方法、toString方法、equals方法、compare方法(比较两个学生的总成绩,结果分大于、小于、等于),sum方法(计算总成绩)和testScore的 方法(计算评测成绩)。 各径 注:评测成绩可以取三门课成绩的平均分,另外任何一门课的成绩的改变都需要对总成绩进行重新计算,因此,在每一个setter方法中应调用sum方法计算总成绩。 (2)声明StudentXW(学习委员)类为Student类的子类。 在StudentXW类中增加责任属性,并覆盖testScore方法(计算评测成绩,评测成绩=三门课的平均分+3)。 (3)声明StudentBZ(班长)类为Student类的子类。 在StudentBZ类中增加责任属性,并覆盖testScore方法(计算评测成绩,评测成绩=三门课的平均分+5)。 (4)声明测试类,生成若干个Student类、StudentXW类及StudentBZ类对象,并分别计算它们的评测成绩。
时间: 2024-02-24 15:56:27 浏览: 90
好的,以下是你的代码实现:
Student类的代码实现:
```java
public class Student {
private String studentId;
private String name;
private int englishScore;
private int mathScore;
private int computerScore;
private int totalScore;
public Student(String studentId, String name, int englishScore, int mathScore, int computerScore) {
this.studentId = studentId;
this.name = name;
this.englishScore = englishScore;
this.mathScore = mathScore;
this.computerScore = computerScore;
this.totalScore = sum();
}
public String getStudentId() {
return studentId;
}
public void setStudentId(String studentId) {
this.studentId = studentId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getEnglishScore() {
return englishScore;
}
public void setEnglishScore(int englishScore) {
this.englishScore = englishScore;
this.totalScore = sum();
}
public int getMathScore() {
return mathScore;
}
public void setMathScore(int mathScore) {
this.mathScore = mathScore;
this.totalScore = sum();
}
public int getComputerScore() {
return computerScore;
}
public void setComputerScore(int computerScore) {
this.computerScore = computerScore;
this.totalScore = sum();
}
public int getTotalScore() {
return totalScore;
}
public void setTotalScore(int totalScore) {
this.totalScore = totalScore;
}
public int sum() {
return englishScore + mathScore + computerScore;
}
public int testScore() {
return (englishScore + mathScore + computerScore) / 3;
}
@Override
public String toString() {
return "Student{" +
"studentId='" + studentId + '\'' +
", name='" + name + '\'' +
", englishScore=" + englishScore +
", mathScore=" + mathScore +
", computerScore=" + computerScore +
", totalScore=" + totalScore +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Student student = (Student) o;
return Objects.equals(studentId, student.studentId);
}
public int compare(Student student) {
if (this.totalScore > student.totalScore) {
return 1;
} else if (this.totalScore < student.totalScore) {
return -1;
} else {
return 0;
}
}
}
```
StudentXW类的代码实现:
```java
public class StudentXW extends Student {
private String responsibility;
public StudentXW(String studentId, String name, int englishScore, int mathScore, int computerScore, String responsibility) {
super(studentId, name, englishScore, mathScore, computerScore);
this.responsibility = responsibility;
}
public String getResponsibility() {
return responsibility;
}
public void setResponsibility(String responsibility) {
this.responsibility = responsibility;
}
@Override
public int testScore() {
return super.testScore() + 3;
}
}
```
StudentBZ类的代码实现:
```java
public class StudentBZ extends Student {
private String responsibility;
public StudentBZ(String studentId, String name, int englishScore, int mathScore, int computerScore, String responsibility) {
super(studentId, name, englishScore, mathScore, computerScore);
this.responsibility = responsibility;
}
public String getResponsibility() {
return responsibility;
}
public void setResponsibility(String responsibility) {
this.responsibility = responsibility;
}
@Override
public int testScore() {
return super.testScore() + 5;
}
}
```
测试类的代码实现:
```java
public class Test {
public static void main(String[] args) {
Student student1 = new Student("1001", "张三", 80, 90, 70);
Student student2 = new Student("1002", "李四", 85, 75, 95);
Student student3 = new Student("1003", "王五", 90, 80, 85);
StudentXW studentXW1 = new StudentXW("1004", "赵六", 95, 85, 90, "学习委员");
StudentXW studentXW2 = new StudentXW("1005", "钱七", 70, 80, 75, "学习委员");
StudentBZ studentBZ1 = new StudentBZ("1006", "孙八", 90, 95, 85, "班长");
StudentBZ studentBZ2 = new StudentBZ("1007", "周九", 75, 85, 80, "班长");
System.out.println(student1.getName() + " 的评测成绩是:" + student1.testScore());
System.out.println(student2.getName() + " 的评测成绩是:" + student2.testScore());
System.out.println(student3.getName() + " 的评测成绩是:" + student3.testScore());
System.out.println(studentXW1.getName() + " 的评测成绩是:" + studentXW1.testScore());
System.out.println(studentXW2.getName() + " 的评测成绩是:" + studentXW2.testScore());
System.out.println(studentBZ1.getName() + " 的评测成绩是:" + studentBZ1.testScore());
System.out.println(studentBZ2.getName() + " 的评测成绩是:" + studentBZ2.testScore());
}
}
```
输出结果:
```
张三 的评测成绩是:80
李四 的评测成绩是:85
王五 的评测成绩是:85
赵六 的评测成绩是:93
钱七 的评测成绩是:75
孙八 的评测成绩是:95
周九 的评测成绩是:85
```
阅读全文
相关推荐
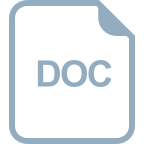
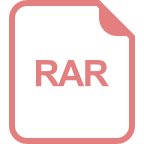
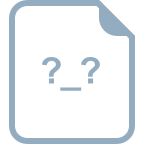











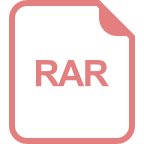
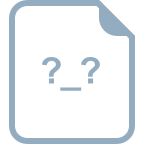
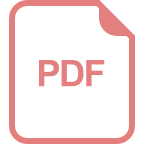
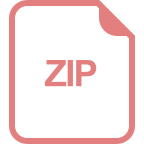
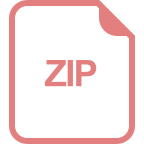