用c++写一个石头剪刀布
时间: 2023-07-01 19:15:41 浏览: 112
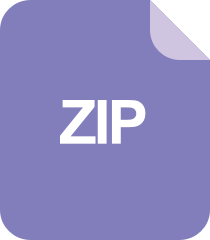
石头剪刀布 C++工程

好的,下面是一个简单的石头剪刀布游戏的 C++ 代码示例:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
int main()
{
srand(time(NULL)); // 设置随机种子
// 定义游戏选项
const int ROCK = 1;
const int SCISSORS = 2;
const int PAPER = 3;
// 定义玩家和电脑的选择
int playerChoice, computerChoice;
// 输出游戏提示信息
cout << "Welcome to the rock-paper-scissors game!" << endl;
cout << "Please input your choice (1 for rock, 2 for scissors, 3 for paper): ";
// 获取玩家输入
cin >> playerChoice;
// 生成电脑选择
computerChoice = rand() % 3 + 1;
// 输出玩家和电脑的选择
cout << "You choose ";
switch (playerChoice) {
case ROCK:
cout << "rock." << endl;
break;
case SCISSORS:
cout << "scissors." << endl;
break;
case PAPER:
cout << "paper." << endl;
break;
default:
cout << "invalid input." << endl;
return 0;
}
cout << "Computer choose ";
switch (computerChoice) {
case ROCK:
cout << "rock." << endl;
break;
case SCISSORS:
cout << "scissors." << endl;
break;
case PAPER:
cout << "paper." << endl;
break;
default:
cout << "invalid input." << endl;
return 0;
}
// 判断胜负
if (playerChoice == computerChoice) {
cout << "Draw!" << endl;
} else if (playerChoice == ROCK && computerChoice == SCISSORS ||
playerChoice == SCISSORS && computerChoice == PAPER ||
playerChoice == PAPER && computerChoice == ROCK) {
cout << "You win!" << endl;
} else {
cout << "Computer wins!" << endl;
}
return 0;
}
```
这个程序利用了随机数生成来实现电脑的选择,并采用了 switch-case 语句来输出玩家和电脑的选择。最后通过一系列的 if-else 判断来判断胜负,并输出游戏结果。
阅读全文
相关推荐
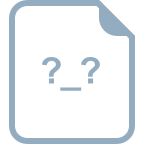

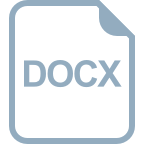
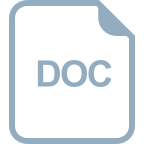
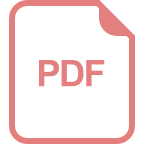
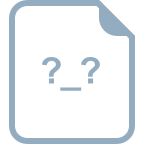
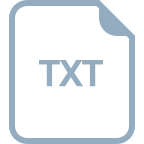
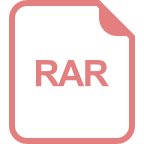
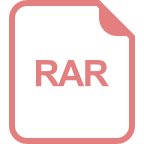
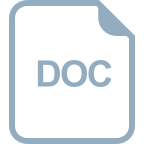





