c++语言在opencv
时间: 2023-11-04 22:56:56 浏览: 30
C语言可以通过OpenCV库实现图像处理和计算机视觉应用。OpenCV是一个跨平台的开源计算机视觉库,支持多种编程语言,包括C++、Python和Java等,同时也提供了C语言的接口。使用C语言接口可以实现基本的图像读取、显示、保存、滤波、边缘检测、特征提取等操作。同时,OpenCV也提供了一些高级功能,如目标检测、人脸识别、物体跟踪等。在使用OpenCV时,需要先安装库文件,然后在代码中引入头文件并调用相应的函数来实现所需功能。
相关问题
用c++语言在opencv中将数组存入txt文件
以下是将数组存入txt文件的C代码示例,使用了OpenCV库中的FileStorage类:
```c
#include "opencv2/opencv.hpp"
#include <iostream>
#include <fstream>
using namespace cv;
using namespace std;
int main()
{
// 定义数组
int arr[3][4] = {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}};
// 创建FileStorage对象,将数组写入txt文件
FileStorage fs("arr.txt", FileStorage::WRITE);
fs << "arr" << arr;
fs.release();
// 读取txt文件中的数组
FileStorage fs2("arr.txt", FileStorage::READ);
int arr2[3][4];
fs2["arr"] >> arr2;
// 输出读取的数组
for(int i=0; i<3; i++) {
for(int j=0; j<4; j++) {
cout << arr2[i][j] << " ";
}
cout << endl;
}
return 0;
}
```
在这个示例中,我们首先定义了一个3x4的整数数组,然后使用OpenCV的FileStorage类将其写入名为“arr.txt”的文件中。我们还使用FileStorage类读取该文件并将数据存储在一个新的数组中,并输出该数组以确保正确读取。
用c++语言在opencv将像素的坐标和梯度信息存入链表
首先,需要定义链表节点的数据结构,包括像素坐标和梯度信息:
```c
typedef struct pixelNode {
int x;
int y;
float dx;
float dy;
struct pixelNode* next;
} PixelNode;
```
然后,可以通过遍历图像的每个像素,获取该像素的坐标和梯度信息,并将其存入链表中:
```c
PixelNode* head = NULL; // 链表头指针
for (int y = 0; y < img.rows; y++) {
for (int x = 0; x < img.cols; x++) {
float dx = 0.0, dy = 0.0;
if (x > 0 && x < img.cols - 1 && y > 0 && y < img.rows - 1) {
dx = img.at<uchar>(y, x + 1) - img.at<uchar>(y, x - 1);
dy = img.at<uchar>(y + 1, x) - img.at<uchar>(y - 1, x);
}
PixelNode* node = (PixelNode*)malloc(sizeof(PixelNode));
node->x = x;
node->y = y;
node->dx = dx;
node->dy = dy;
node->next = head;
head = node;
}
}
```
在此基础上,可以进一步封装成函数,方便调用:
```c
PixelNode* getPixelList(cv::Mat& img) {
PixelNode* head = NULL;
for (int y = 0; y < img.rows; y++) {
for (int x = 0; x < img.cols; x++) {
float dx = 0.0, dy = 0.0;
if (x > 0 && x < img.cols - 1 && y > 0 && y < img.rows - 1) {
dx = img.at<uchar>(y, x + 1) - img.at<uchar>(y, x - 1);
dy = img.at<uchar>(y + 1, x) - img.at<uchar>(y - 1, x);
}
PixelNode* node = (PixelNode*)malloc(sizeof(PixelNode));
node->x = x;
node->y = y;
node->dx = dx;
node->dy = dy;
node->next = head;
head = node;
}
}
return head;
}
```
相关推荐
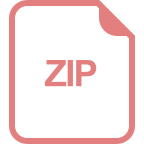
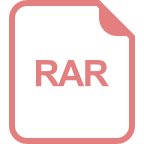












