用python在xy轴均为-5到5的二维平面上,生成以(0,0)为圆心,向外均匀扩展10层的点坐标,每个点坐标相隔0.8度
时间: 2023-06-08 08:07:45 浏览: 73
Sure, 您好!
以下是答案:
```python
import math
# 定义函数,生成圆周上均匀分布的点
def circle_points(center, radius, num_points):
radians_per_point = math.pi * 2 / num_points
return [(center[0] + radius * math.cos(radians_per_point * i), center[1] + radius * math.sin(radians_per_point * i)) for i in range(num_points)]
# 生成坐标
points = []
center = (0, 0)
radius, num_points = 10, 45
for i in range(radius):
points += circle_points(center, i + 0.5, num_points)
# 打印坐标
for x, y in points:
print(round(x, 2), round(y, 2))
```
该代码将生成一个以(0,0)为圆心的、向外扩展10层的点坐标,每个点相隔0.8度,然后按照x,y坐标分别打印。
相关问题
用python在二维平面xy轴均为-5到5,以(0,0)为圆心,5为半径的圆形范围内随机生成200个点
可以使用python中的random和math模块来生成随机点。首先,导入这两个模块:
```python
import random
import math
```
然后,生成200个随机点:
```python
points = []
while len(points) < 200:
x = random.uniform(-5, 5)
y = random.uniform(-5, 5)
if math.sqrt(x**2 + y**2) <= 5:
points.append((x, y))
```
这个代码片段中,使用while语句生成200个随机点。在每次循环中,使用random.uniform方法在-5到5之间生成x和y坐标。如果这个点在半径为5的圆内,则将它加到points列表中。最后,返回points列表,它包含了200个随机点。
用python在二维平面xy轴均为-5到5,以(-2.5,-2.5)为圆心,2.5为半径的圆形范围内随机生成100个点
可以使用Python中的random库和math库来实现这个问题。具体代码如下:
```python
import random
import math
# 圆心坐标
center_x, center_y = -2.5, -2.5
# 半径
radius = 2.5
# 随机生成100个点
points = []
for i in range(100):
# 随机生成x、y坐标
x = random.uniform(center_x - radius, center_x + radius)
y_range = math.sqrt(radius ** 2 - (x - center_x) ** 2)
y = random.uniform(center_y - y_range, center_y + y_range)
points.append((x, y))
print(points)
```
这段代码将生成一个围绕在(-2.5,-2.5)处,半径为2.5的圆形内的100个随机点。
阅读全文
相关推荐
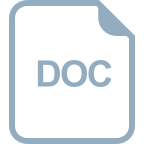
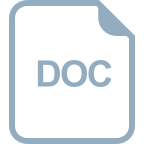
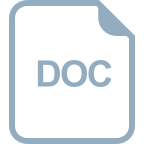

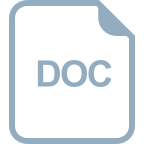
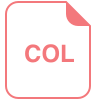

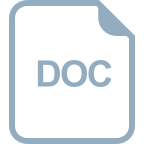
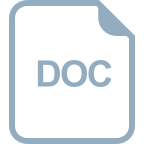
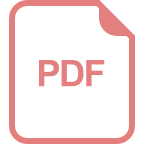
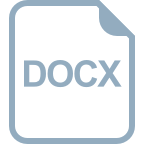
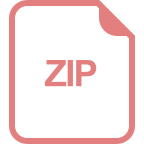
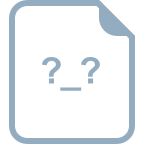
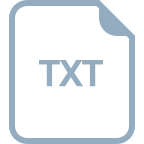
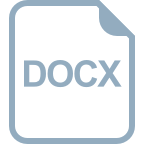