python实现室内机器人五次多项式轨迹规划
时间: 2023-08-09 11:01:09 浏览: 99
Python实现室内机器人五次多项式轨迹规划可以通过使用numpy库进行实现。以下是一个简单的示例代码:
```python
import numpy as np
def quintic_polynomial_trajectory(start_pos, end_pos, start_vel, end_vel, start_acc, end_acc, duration, num_points):
time = np.linspace(0, duration, num_points)
# 通过求解五次多项式的系数来得到轨迹
A = np.array([[0, 0, 0, 0, 0, 1],
[duration**5, duration**4, duration**3, duration**2, duration, 1],
[0, 0, 0, 0, 1, 0],
[5*duration**4, 4*duration**3, 3*duration**2, 2*duration, 1, 0],
[0, 0, 0, 2, 0, 0],
[20*duration**3, 12*duration**2, 6*duration, 2, 0, 0]])
b_x = np.array([start_pos[0], end_pos[0], start_vel[0], end_vel[0], start_acc[0], end_acc[0]])
b_y = np.array([start_pos[1], end_pos[1], start_vel[1], end_vel[1], start_acc[1], end_acc[1]])
b_z = np.array([start_pos[2], end_pos[2], start_vel[2], end_vel[2], start_acc[2], end_acc[2]])
# 使用线性方程组求解得到轨迹中每个时间点的位置
x_coeffs = np.linalg.solve(A, b_x)
y_coeffs = np.linalg.solve(A, b_y)
z_coeffs = np.linalg.solve(A, b_z)
traj = np.zeros((num_points, 3))
for i in range(num_points):
t = np.array([1, time[i], time[i]**2, time[i]**3, time[i]**4, time[i]**5])
traj[i] = np.array([np.dot(x_coeffs, t), np.dot(y_coeffs, t), np.dot(z_coeffs, t)])
return traj
```
使用该函数可以得到室内机器人在给定起始位置、终点位置、起始速度、终点速度、起始加速度、终点加速度、持续时间和轨迹点数的条件下,生成的五次多项式轨迹。这样的轨迹规划可用于室内机器人的自主移动、路径规划和动作控制等任务。
相关推荐
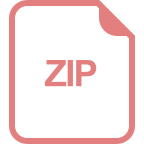














