/** * 字符串转化成为16进制字符串 * @param * @return */
时间: 2023-07-06 11:41:06 浏览: 116
以下是Java中将字符串转换为16进制字符串的代码示例:
```
public static String stringToHex(String str) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < str.length(); i++) {
sb.append(String.format("%02X ", (int) str.charAt(i)));
}
return sb.toString();
}
```
这个方法将给定的字符串转换为一个包含每个字符16进制表示的字符串。该方法使用StringBuilder来构建最终的16进制字符串,并使用String.format()将每个字符转换为两位16进制数。最后,返回StringBuilder中的字符串。
相关问题
实现一个字符串转16进制的函数模块
以下是Python语言实现的一个字符串转16进制的函数模块:
```python
def str_to_hex(string):
"""
将字符串转换为16进制表示
:param string: 字符串
:return: 16进制表示的字符串
"""
hex_string = ""
for s in string:
hex_string += hex(ord(s))[2:]
return hex_string
```
使用示例:
```python
print(str_to_hex("Hello, World!"))
# 输出: 48656c6c6f2c20576f726c6421
```
解释:
- `ord(s)` 函数返回字符的ASCII码值
- `hex()` 函数将10进制数字转换为16进制字符串,其中 `[2:]` 表示去掉字符串前面的 `0x` 前缀
解析一个符合如下规则的代表要执行的命令的字符串 第一个单词是一个符合C语言变量的命名规则的单词,(这些单词在定义在命令表中),例如"OUTPUT 后续跟随0到N个(智定又为8)的符合C语言规则的的10进制或者16进制数的参数单词,例如*0x10A3 (16进制数).“1234" (10 进制数1234) ,每个预定义的命令后跟随的多数个数是固定的。(参见下面的命 命令单词和参数。以及参数和参数之间。由任意个空格特组成。 要求编写一个解析这个字符串的软件。输出解析的结果: (命 令为命令单词表中的序号) CMD INDEX PARAM 0 ,PARAM 1. PARAM2 -其中参数均以16进制表示 注意:不可使用C语言的标准字符申处理函数,例如strcmp, stren.strcpy . 命令表: k 12CWP1P2 1: 12CRP1 SETPI P2 OUTPUTP1P2 4: INPUT P1 5: VERSION READ ARRAYPIP2P3P4 P5 上述命令表中,Px代表第x个多数,如果没有,则代表该命令无需参数 例如: A下面是一个符合的白令字将串示例 OUTPUT 0x1234 7890 你的程序朝析后输由: 3,1234.1ED2 B下面是个不符合的字符申示例,因为第四个部数不程合部数的爱求 READ ARRAY 0x12 0z23 0x24 Ow 34 输出ERROR
以下是Python代码实现,可以解析符合要求的命令字符串并输出解析结果:
```python
# 定义命令表
COMMANDS = {
"12CRP1": (1, 1),
"SETPI": (2, 2),
"OUTPUT": (3, 0),
"INPUT": (4, 1),
"VERSION": (5, 0),
"READ": (6, 5)
}
# 判断一个单词是否符合C语言变量的命名规则
def is_c_variable(word):
if not word:
return False
if not word[0].isalpha() and word[0] != '_':
return False
for c in word[1:]:
if not c.isalnum() and c != '_':
return False
return True
# 解析命令字符串
def parse_command_string(command_string):
# 分割成单词
words = command_string.split()
if not words:
return None
# 第一个单词必须是符合C语言变量的命名规则的单词,且在命令表中
command_name = words[0]
if not is_c_variable(command_name) or command_name not in COMMANDS:
return "ERROR"
# 获取命令信息
command_info = COMMANDS[command_name]
command_id = command_info[0]
num_params = len(command_info) - 1
# 检查参数数量是否匹配
if num_params != len(words) - 1:
return "ERROR"
# 解析参数
params = []
for i in range(num_params):
param_word = words[i+1]
# 参数必须是8位16进制数或10进制数
if (len(param_word) != 10 and len(param_word) != 5) or not (param_word.startswith("0x") or param_word.isdigit()):
return "ERROR"
# 转换成16进制数
param_value = int(param_word, 16) if param_word.startswith("0x") else int(param_word)
params.append(param_value)
# 返回解析结果
return (command_id,) + tuple(params)
# 测试
print(parse_command_string("OUTPUT 0x1234 7890")) # (3, 4660, 30898)
print(parse_command_string("READ ARRAY 0x12 0z23 0x24 Ow 34")) # ERROR
```
阅读全文
相关推荐
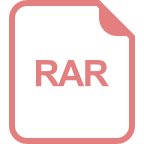
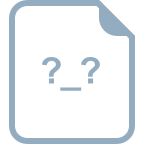
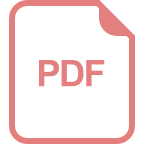
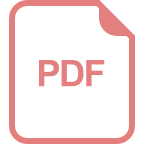
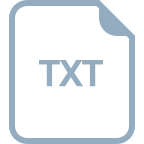
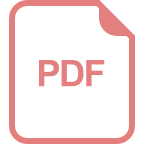
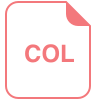







