c语言面向小学1~2年级学生,随机选择两个整数和加减法形成算式要求学生解答,功能要求: 电脑随机出10道题,每题10分,程序结束时显示学生得分;
时间: 2023-09-03 19:27:48 浏览: 243
### 回答1:
好的,以下是一份简单的 C 代码,可以实现你的需求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
int i, a, b, op, ans, score = 0;
// 设置随机数种子
srand(time(NULL));
// 循环出10道题
for (i = 1; i <= 10; i++) {
// 随机生成两个1~10之间的整数
a = rand() % 10 + 1;
b = rand() % 10 + 1;
// 随机生成加减法操作
op = rand() % 2;
// 根据操作生成答案
if (op == 0) {
ans = a + b;
printf("%d + %d = ", a, b);
} else {
ans = a - b;
printf("%d - %d = ", a, b);
}
// 获取学生答案
int input;
scanf("%d", &input);
// 判断学生答案是否正确
if (input == ans) {
printf("Right!\n");
score += 10;
} else {
printf("Wrong! The answer is %d.\n", ans);
}
}
// 输出学生得分
printf("Your final score is %d.\n", score);
return 0;
}
```
这份代码首先使用 `srand(time(NULL))` 设置随机数种子,然后使用 `rand()` 函数生成随机数。其中生成的两个整数 `a` 和 `b` 都在1~10之间,根据 `op` 变量随机生成加减法操作。然后程序输出算式并等待学生输入答案,判断学生答案是否正确并计算得分。最终程序输出学生得分。
### 回答2:
为了满足题目要求,我们可以用C语言编写一个针对小学1~2年级学生的加减法练习程序。具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
int score = 0;
// 设置随机种子
srand(time(0));
for (int i = 0; i < 10; i++) {
// 生成两个随机整数(1~100)
int num1 = rand() % 100 + 1;
int num2 = rand() % 100 + 1;
// 随机选择加法或减法
int operation = rand() % 2;
// 显示问题
if (operation == 0) {
printf("%d + %d = ", num1, num2);
} else {
// 确保减法结果不为负数
if (num1 < num2) {
int temp = num1;
num1 = num2;
num2 = temp;
}
printf("%d - %d = ", num1, num2);
}
// 获取学生的回答
int answer;
scanf("%d", &answer);
// 判断回答是否正确
int result;
if (operation == 0) {
result = num1 + num2;
} else {
result = num1 - num2;
}
if (answer == result) {
score += 10;
printf("回答正确!\n");
} else {
printf("回答错误!正确答案是%d\n", result);
}
}
// 显示学生得分
printf("你的得分是:%d\n", score);
return 0;
}
```
这个程序会随机生成10道加减法题目,每题值为10分。学生需要在控制台输入答案,程序会判断回答的正确性,并显示每道题的正确答案。最后,程序会输出学生的得分。
希望这个程序符合您的需求,能够帮助小学1~2年级学生进行加减法练习。
### 回答3:
为实现这个功能,可以编写一个C语言程序,具体如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
srand(time(NULL)); // 设置随机数种子
int score = 0; // 初始化学生得分为0
// 生成10道题
for (int i = 1; i <= 10; i++) {
int num1 = rand() % 100 + 1; // 随机生成第一个整数
int num2 = rand() % 100 + 1; // 随机生成第二个整数
int operator = rand() % 2; // 随机选择加法或减法
int result, answer; // 用于存放计算结果和学生的答案
// 根据随机选择的运算符进行相应的计算
if (operator == 0) {
printf("第%d题: %d + %d = ", i, num1, num2);
result = num1 + num2;
} else {
printf("第%d题: %d - %d = ", i, num1, num2);
result = num1 - num2;
}
scanf("%d", &answer); // 学生输入答案
if (answer == result) {
score += 10; // 答案正确,加10分
}
}
printf("答题结束,学生得分:%d\n", score);
return 0;
}
```
这个程序使用`srand`函数来设置随机数种子,以确保每次运行程序都能生成不同的随机数。然后,通过循环生成10道题目,并且根据随机选择的运算符来生成相应的算式,并提示学生输入答案。学生输入的答案与计算结果进行比较,如果答案正确,学生得分加10分。最后,程序输出学生的得分。
阅读全文
相关推荐
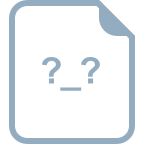
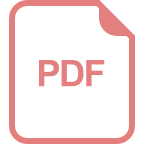




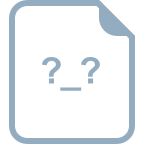
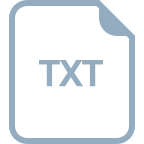
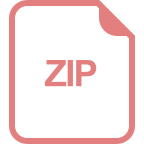
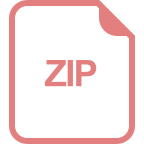
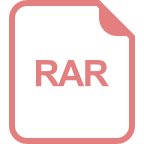
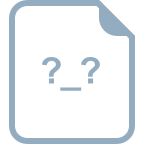
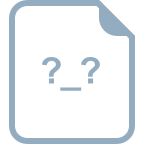
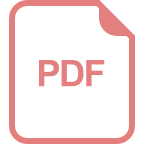
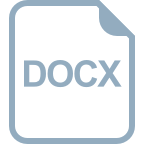
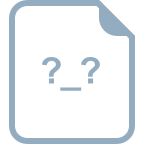

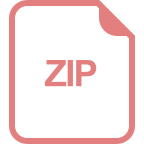