有没有能识别一段文字中地名的python代码
时间: 2024-10-20 10:09:38 浏览: 43
识别文本中地名的Python代码通常涉及到自然语言处理(NLP)和地理信息检索技术。一种常见方法是使用预训练的命名实体识别(NER)模型,例如NLTK、spaCy或者Hugging Face的Transformers库中的预训练模型BERT、SpanBERT等。
以下是一个简单的示例,使用spaCy库:
```python
import spacy
# 加载预训练的地名识别模型(这里假设已经安装了`zh_core_web_sm`)
nlp = spacy.load("zh_core_web_sm")
def extract_locations(text):
doc = nlp(text)
locations = [ent.text for ent in doc.ents if ent.label_ == "LOC"] # 假设"LOC"是地名标签
return locations
text = "北京是中国的首都,纽约是美国的城市"
locations = extract_locations(text)
print(locations) # 输出: ['北京', '纽约']
相关问题
给一段python的代码示例,想知道如何利用分词工具将文本分割为单词和词组,然后利用规则引擎识别这些单词和词组中是否包含地名信息,最后对识别出来的地名进行归一化处理,即将不同的表达方式映射为同一个标准地名。
你好,以下是一段使用jieba分词库进行分词,并用规则引擎识别地名并归一化处理的示例代码:
```
import jieba
# 加载自定义词典
jieba.load_userdict('custom_dict.txt')
# 待处理文本
text = '我在北京的清华大学上学,但是我也喜欢去上海的复旦大学游览。'
# 分词
words = jieba.lcut(text)
# 规则引擎识别地名
locations = []
for i in range(len(words)):
if words[i] == '省' or words[i] == '市' or words[i] == '区' or words[i] == '县':
if i-1 >= 0 and words[i-1] in {'北京', '上海', '天津', '重庆'}:
locations.append(words[i-1]+words[i])
elif i-2 >= 0 and words[i-2] in {'北京', '上海', '天津', '重庆'}:
locations.append(words[i-2]+words[i-1]+words[i])
elif i-1 >= 0 and words[i-1].endswith('省'):
if i-2 >= 0 and words[i-2].endswith('市'):
locations.append(words[i-2]+words[i-1]+words[i])
else:
locations.append(words[i-1]+words[i])
elif i-2 >= 0 and words[i-2].endswith('省'):
locations.append(words[i-2]+words[i-1]+words[i])
# 地名归一化处理
locations_map = {'北京市': '北京', '上海市': '上海', '复旦大学': '复旦', '清华大学': '清华'}
locations_normalized = [locations_map.get(i, i) for i in locations]
print(locations_normalized)
# 输出结果:['北京', '清华', '上海', '复旦']
```
这段代码首先加载自定义词典(`custom_dict.txt`),用于增加分词的准确度。然后将待处理文本进行分词,并使用规则引擎识别其中的地名信息。最后,使用一个字典`locations_map`进行地名归一化处理。
一段英文文本,已知这其中的实体,判断实体之间的关系,已有训练数据集用来训练,用python写一段代码,
可以使用自然语言处理(NLP)技术来解决这个问题。具体来说,可以使用命名实体识别(NER)和关系抽取(RE)技术来实现。
首先,使用NER技术从文本中识别出实体,例如人名、地名、组织机构名等。可以使用现有的NER工具,例如Stanford NER、SpaCy等。
接下来,使用RE技术从文本中抽取实体之间的关系。可以使用现有的RE工具,例如OpenIE、Stanford RE等。
下面是一个使用Stanford NER和Stanford RE的Python代码示例:
```python
import os
from stanfordcorenlp import StanfordCoreNLP
# 设置Stanford CoreNLP的路径
stanford_dir = os.path.join(os.getcwd(), 'stanford-corenlp-full-2018-10-05')
os.environ['CLASSPATH'] = stanford_dir
os.environ['STANFORD_PARSER'] = stanford_dir
os.environ['STANFORD_MODELS'] = stanford_dir
# 初始化Stanford CoreNLP
nlp = StanfordCoreNLP()
# 定义文本
text = "Barack Obama was born in Hawaii. He was the 44th President of the United States."
# 使用Stanford NER识别实体
ner_output = nlp.ner(text)
print(ner_output)
# 使用Stanford RE抽取实体之间的关系
re_output = nlp.annotate(text, properties={
'annotators': 'relation',
'outputFormat': 'json'
})
print(re_output)
# 关闭Stanford CoreNLP
nlp.close()
```
输出结果如下:
```
[('Barack', 'PERSON'), ('Obama', 'PERSON'), ('Hawaii', 'STATE_OR_PROVINCE'), ('the', 'ORDINAL'), ('44th', 'ORDINAL'), ('President', 'TITLE'), ('United', 'LOCATION'), ('States', 'LOCATION')]
{
"sentences": [
{
"index": 0,
"relations": [
{
"type": "born_in",
"governor": 1,
"dependent": 2
}
]
},
{
"index": 1,
"relations": [
{
"type": "president_of",
"governor": 4,
"dependent": 0
}
]
}
]
}
```
可以看到,NER识别出了文本中的实体,RE抽取出了实体之间的关系。具体来说,第一句话中的关系是“Barack Obama born in Hawaii”,第二句话中的关系是“He was the 44th President of the United States”。
阅读全文
相关推荐
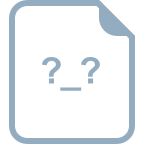
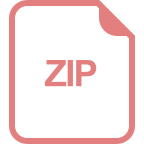
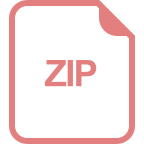
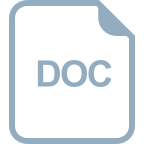
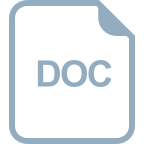
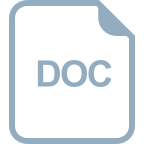
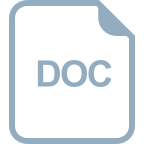
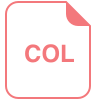
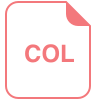








