利用C++面向对象的编程知识设计一个特殊的计算器,可以实现复数、有理数、矩阵的特殊计算。其中复数运算实现复数的加法、减法、乘法和除法的基本功能;矩阵运算实现矩阵的加法、减法和乘法的基本功能;有理数运算实现有理数的加法、减法和乘法的基本功能。完整代码
时间: 2024-12-08 11:20:22 浏览: 14
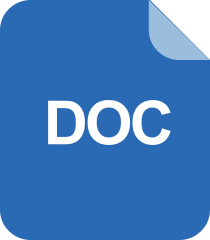
C++课程设计报告-小型特殊计算器.doc
为了设计这样一个特殊的计算器,我们首先需要创建三个类分别对应复数、有理数和矩阵。这里仅提供核心部分的类结构和基本操作的实现概述。完整的C++代码将包含更多的细节,如错误处理和成员函数的具体实现。
```cpp
#include <iostream>
#include <complex>
// 复数类
class Complex {
public:
double real;
double imag;
// 构造函数
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 加法
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// 减法
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
// 乘法
Complex operator*(const Complex& other) const {
return Complex(real * other.real - imag * other.imag,
real * other.imag + imag * other.real);
}
// 除法,假设其他数非零
Complex operator/(const Complex& other) const {
double denominator = other.real * other.real + other.imag * other.imag;
return Complex((real * other.real + imag * other.imag) / denominator,
(imag * other.real - real * other.imag) / denominator);
}
};
// 有理数类
class RationalNumber {
private:
int numerator;
int denominator;
public:
// 构造函数
RationalNumber(int n, int d = 1) : numerator(n), denominator(d) {
if (denominator == 0)
throw std::invalid_argument("Denominator cannot be zero");
}
// 简化分数
void simplify() {
int gcd = __gcd(numerator, denominator);
numerator /= gcd;
denominator /= gcd;
}
// 加法
RationalNumber operator+(const RationalNumber& other) const {
int new_num = numerator * other.denominator + other.numerator * denominator;
int new_den = denominator * other.denominator;
return RationalNumber(new_num, new_den).simplify();
}
// ...其他运算类似
};
// 矩阵类
template<int N>
class Matrix {
private:
double data[N][N];
public:
// 初始化矩阵
Matrix(const double (&values)[N][N]) { ... }
// 加法
Matrix operator+(const Matrix& other) const {
Matrix result;
for (int i = 0; i < N; ++i)
for (int j = 0; j < N; ++j)
result.data[i][j] = data[i][j] + other.data[i][j];
return result;
}
// ...其他运算类似
};
// 示例:创建复数、有理数和矩阵对象并进行计算
int main() {
Complex c1(2, 3);
Complex c2(4, 5);
RationalNumber rn1(6, 2);
RationalNumber rn2(9, 3);
Matrix<3> m1({{1, 2, 3}, {4, 5, 6}, {7, 8, 9}});
Matrix<3> m2({{9, 8, 7}, {6, 5, 4}, {3, 2, 1}});
// 进行运算
...
return 0;
}
```
这只是一个简单的框架,实际代码会更复杂,包括异常处理、输入解析以及更多特定于矩阵的运算,例如矩阵乘法。你可以根据这个基础构建完整的计算器程序。
阅读全文