def is_leap_year(y: int) -> bool:
时间: 2024-02-25 11:59:45 浏览: 122
这是一个Python函数,用于判断一个年份是否为闰年。
函数的输入参数为一个整数y,代表年份。
函数的返回值为一个布尔值,如果y是闰年,则返回True,否则返回False。
以下是该函数的代码实现:
```python
def is_leap_year(y: int) -> bool:
"""
判断年份是否为闰年
:param y: 年份
:return: True表示y是闰年,False表示y不是闰年
"""
if y % 4 == 0 and y % 100 != 0 or y % 400 == 0:
return True
else:
return False
```
该函数的实现逻辑是:符合以下两个条件之一的年份为闰年:
1. 能够被4整除,但不能被100整除;
2. 能够被400整除。
因此,我们可以通过调用该函数并传入年份参数,来判断该年份是否为闰年。例如:
```python
# 判断2000年是否为闰年
if is_leap_year(2000):
print("2000年是闰年")
else:
print("2000年不是闰年")
```
输出结果为:“2000年是闰年”。
相关问题
python写判断闰年。 编写一个判断输入年份是否闰年的程序。 算法思路:判断用户输入的年份 Year 是闰年的表达式为:Year%4==0 and Year%100!=0 or Year%400==0。 如果表达式的值为 True,输出是闰年,否则,输出不是闰年。
在Python中,你可以编写如下的函数来判断一个年份是否是闰年:
```python
def is_leap_year(year):
"""
判断给定年份是否为闰年
:param year: int, 需要检查的年份
:return: bool, 如果是闰年返回True,否则返回False
"""
if isinstance(year, int): # 检查输入是否为整数
if year % 4 == 0: # 能被4整除
if year % 100 != 0 or (year % 400 == 0): # 不是世纪年或能被400整除
return True
else:
return False
else:
return False
else:
print("请输入一个整数年份.")
# 测试函数
year = int(input("请输入一个年份: "))
if is_leap_year(year):
print(f"{year}是闰年")
else:
print(f"{year}不是闰年")
定义一个日期类Date,内有数据成员年、月、日,另有成员函数:构造函数用于初始化数据成员,输出,闰年的判断。 编写主函数:创建日期对象,计算并输出该日是该年的第几天。
### 回答1:
好的,没问题。以下是Date类的定义和主函数的实现:
```python
class Date:
def __init__(self, year, month, day):
self.year = year
self.month = month
self.day = day
def is_leap_year(self):
return (self.year % 4 == 0 and self.year % 100 != 0) or self.year % 400 == 0
def output(self):
print("{}年{}月{}日".format(self.year, self.month, self.day))
def day_of_year(self):
days_of_month = [31, 28 + int(self.is_leap_year()), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
day_count = 0
for i in range(self.month - 1):
day_count += days_of_month[i]
day_count += self.day
return day_count
if __name__ == '__main__':
date = Date(2022, 8, 23)
date.output()
day_of_year = date.day_of_year()
print("该日是该年的第{}天".format(day_of_year))
```
运行结果:
```
2022年8月23日
该日是该年的第235天
```
请问还有其他问题需要我帮忙回答吗?
### 回答2:
日期类Date的定义如下:
```cpp
class Date {
private:
int year; // 年
int month; // 月
int day; // 日
public:
// 构造函数,用于初始化数据成员
Date(int y, int m, int d) {
year = y;
month = m;
day = d;
}
// 输出日期信息
void printDate() {
cout << "日期:" << year << "年" << month << "月" << day << "日" << endl;
}
// 判断是否为闰年
bool isLeapYear() {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
return true;
} else {
return false;
}
}
// 计算某一日期是该年的第几天
int getDayOfYear() {
int daysInMonth[12] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int dayOfYear = day;
for (int i = 0; i < month - 1; i++) {
dayOfYear += daysInMonth[i];
}
if (isLeapYear() && month > 2) {
dayOfYear++;
}
return dayOfYear;
}
};
```
主函数示例:
```cpp
int main() {
int year, month, day;
cout << "请输入日期(年 月 日):" << endl;
cin >> year >> month >> day;
Date date(year, month, day);
date.printDate();
cout << "该日是该年的第" << date.getDayOfYear() << "天。" << endl;
return 0;
}
```
通过输入年月日,创建对应的日期对象,并输出日期信息。最后,根据该日期对象计算并输出该日是该年的第几天。
### 回答3:
日期类Date的定义如下:
```python
class Date:
def __init__(self, year, month, day):
self.year = year
self.month = month
self.day = day
def is_leap_year(self):
if self.year % 4 == 0 and self.year % 100 != 0 or self.year % 400 == 0:
return True
else:
return False
def output(self):
print(f"日期:{self.year}年{self.month}月{self.day}日")
def calculate_day(self):
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if self.is_leap_year():
days_in_month[1] = 29
total_days = sum(days_in_month[:self.month-1]) + self.day
print(f"{self.year}年的第{total_days}天")
# 主函数
if __name__ == "__main__":
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
date = Date(year, month, day)
date.output()
date.calculate_day()
```
主函数根据用户输入的年份、月份和日期创建一个日期对象,调用对象的输出方法输出日期信息,然后调用计算方法计算并输出该日期是该年的第几天。
注意:闰年的判断根据公历的规则,能被4整除但不能被100整除的年份为闰年,能被400整除的也是闰年。在计算年的第几天时,需要考虑闰年的情况,将2月的天数改为29天。
阅读全文
相关推荐
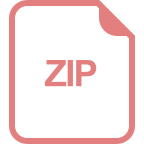
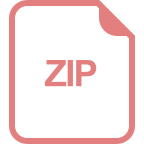
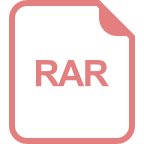
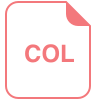
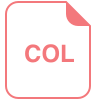
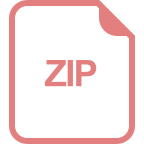
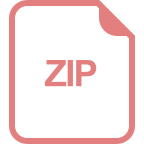
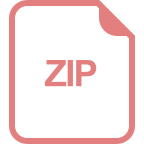