函数is_leap_year判断输入的数是否为闰年
时间: 2024-04-29 20:25:13 浏览: 142
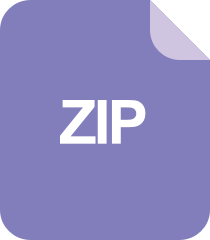
runnian.zip_闰年_闰年判断
好的,函数is_leap_year可以用以下代码实现:
```python
def is_leap_year(year):
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
return True
else:
return False
```
这个函数可以判断输入的年份是否为闰年。如果是闰年,返回True,否则返回False。
阅读全文
相关推荐
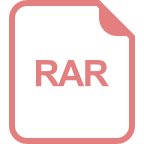
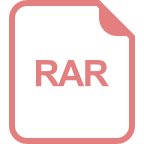















