winfrom学籍管理系统+SQL Server数据库实现多表查询;显示学生姓名,学号,身份证信息,班级,所属学院,专业名称;具体实现过程以及代码
时间: 2024-03-12 22:44:01 浏览: 21
好的,要实现WinForm学籍管理系统+SQL Server数据库实现多表查询,显示学生姓名、学号、身份证信息、班级、所属学院、专业名称,具体实现过程如下:
1. 数据库设计:需要设计学生表、班级表、学院表、专业表等,通过主键和外键进行表之间的关联。
2. 数据库连接:在WinForm应用程序中,需要连接SQL Server数据库,可以使用System.Data.SqlClient命名空间中的类进行连接。
3. 多表查询:在WinForm应用程序中,可以使用SQL语句进行多表查询,例如:
```
SELECT student.name, student.sid, student.idcard, class.classname, college.collegename, major.majorname
FROM student
JOIN class ON student.classid = class.id
JOIN major ON class.majorid = major.id
JOIN college ON major.collegeid = college.id
```
4. 数据显示:在WinForm应用程序中,可以使用DataGridView控件显示查询结果。可以将查询结果绑定到DataGridView控件上,然后设置DataGridView列的HeaderText属性为学生姓名、学号、身份证信息、班级、所属学院、专业名称,并设置DataGridView的数据源为查询结果。
下面是一个示例代码:
```csharp
using System;
using System.Data;
using System.Data.SqlClient;
using System.Windows.Forms;
namespace StudentManagementSystem
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnQuery_Click(object sender, EventArgs e)
{
string connectionString = "Data Source=(local);Initial Catalog=StudentDB;Integrated Security=True";
string sql = "SELECT student.name, student.sid, student.idcard, class.classname, college.collegename, major.majorname " +
"FROM student " +
"JOIN class ON student.classid = class.id " +
"JOIN major ON class.majorid = major.id " +
"JOIN college ON major.collegeid = college.id";
SqlDataAdapter adapter = new SqlDataAdapter(sql, connectionString);
DataTable table = new DataTable();
adapter.Fill(table);
dataGridView1.DataSource = table;
dataGridView1.Columns[0].HeaderText = "学生姓名";
dataGridView1.Columns[1].HeaderText = "学号";
dataGridView1.Columns[2].HeaderText = "身份证信息";
dataGridView1.Columns[3].HeaderText = "班级";
dataGridView1.Columns[4].HeaderText = "所属学院";
dataGridView1.Columns[5].HeaderText = "专业名称";
}
}
}
```
在上面的代码中,btnQuery_Click事件处理程序中,首先定义了连接字符串和SQL语句,然后创建了SqlDataAdapter对象,并使用Fill方法填充查询结果到DataTable中,最后将DataTable设置为DataGridView的数据源,并设置列的HeaderText属性。
相关推荐
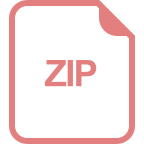
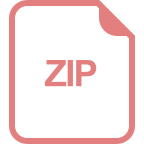
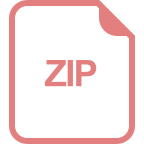














