Python将文本存到txt
时间: 2023-03-19 18:24:02 浏览: 102
在Python中将文本存储到txt文件中可以使用内置的`open()`函数。以下是一个简单的示例代码:
```python
# 打开或创建一个名为myfile.txt的文本文件,并将文本写入其中
with open('myfile.txt', 'w') as f:
f.write('Hello, world!\n')
f.write('This is a text file created by Python.\n')
```
在这个示例中,`open()`函数打开或创建一个名为`myfile.txt`的文件,并使用`'w'`模式打开文件以进行写入。我们使用`with`语句来自动关闭文件,以确保在程序完成写入操作后,文件句柄正确地关闭。
我们使用`f.write()`函数将文本写入文件。每次调用`f.write()`函数时,都会将传递给函数的文本添加到文件中。在文本末尾添加`\n`表示我们要将文本写入一个新行。
当程序执行完`with`语句块时,文件将自动关闭,并保存我们写入的文本。现在,您可以在文件系统中查看`myfile.txt`文件,并看到它包含了我们写入的文本。
相关问题
python如何读入一个txt文本,存成dataFrame
要读取一个txt文本并将其存储为DataFrame,可以使用pandas库中的read_csv函数。以下是一个示例代码:
```
import pandas as pd
df = pd.read_csv('example.txt', delimiter='\t', header=None)
```
在这个例子中,我们假设要读取的txt文件名为example.txt,它使用制表符作为分隔符,并且没有列名。如果你的txt文件使用其他分隔符或者有列名,你需要相应地调整delimiter和header参数。
python批处理存txt文件
使用Python进行批处理存储txt文件是一种常见的操作。可以使用os库来进行文件夹的创建和文件的写入。首先需要导入os库,并使用os.makedirs()函数创建一个文件夹。然后使用open()函数以'w'模式打开一个文件,并使用write()函数将内容写入文件。以下是一个示例代码:
```
import os
# 创建文件夹
os.makedirs('txt_files')
# 批量创建txt文件
for i in range(10):
file_name = f'test_{i}.txt'
file_path = os.path.join('txt_files', file_name)
with open(file_path, 'w') as f:
f.write('This is a test file.')
# 检查文件是否创建成功
files = os.listdir('txt_files')
print(files)
```
以上代码会在当前目录下创建一个名为"txt_files"的文件夹,并在该文件夹下创建10个名为"test_0.txt"到"test_9.txt"的txt文件,并将内容写入文件中。最后,使用os.listdir()函数检查文件是否创建成功。
请注意,这只是一个简单的示例,具体的操作可能因具体需求而有所不同。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [Python批量处理文件的方法总结(包括folder、txt、xml、excel)](https://blog.csdn.net/qq_44701243/article/details/120038287)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *3* [python批量读取txt文本,处理,并批量保存](https://blog.csdn.net/qq_34904125/article/details/123120473)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
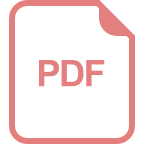
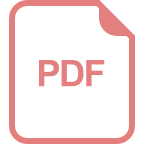
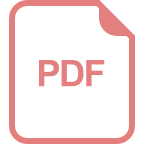
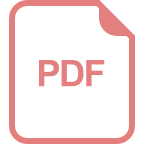









