c++builder 调用调用和生成时为什么需要加__stdcall
时间: 2023-12-17 15:04:32 浏览: 116
在 C++ 中,函数调用约定指定了编译器如何在函数调用时传递参数、返回值、以及如何清理函数栈。C++Builder 默认的函数调用约定是 __cdecl,这种约定要求调用者清理函数栈。
而在 Windows 系统中,许多 API 函数采用的是 __stdcall 调用约定,这种约定要求被调用者清理函数栈。如果在调用 API 函数时使用了错误的调用约定,可能会导致程序崩溃或产生异常。
因此,在使用 C++Builder 开发 Windows 应用程序时,如果需要调用 Windows API 函数,就需要指定函数调用约定为 __stdcall。在函数声明和实现时,需要使用 __stdcall 关键字来指定函数调用约定,例如:
```c++
int __stdcall MyFunction(int arg1, int arg2)
{
// 函数实现
}
```
这样,在调用 MyFunction 函数时,就会使用 __stdcall 调用约定,确保与 Windows API 函数的调用方式一致,避免出现不必要的错误。
相关问题
c++builder 调用和生成dll时为什么需要加__stdcall
在 C++ 中,函数调用约定指定了编译器如何在函数调用时传递参数、返回值、以及如何清理函数栈。在 Windows 平台上,许多 API 函数采用的是 __stdcall 调用约定,这种约定要求被调用者清理函数栈。如果在调用 API 函数时使用了错误的调用约定,可能会导致程序崩溃或产生异常。
因此,在使用 C++Builder 开发 Windows 应用程序时,如果需要编写一个可以被其他程序调用的 DLL,就需要将 DLL 中的函数调用约定指定为 __stdcall,以便其他程序正确调用。
在 C++Builder 中,可以使用 `__declspec(dllexport)` 关键字来导出 DLL 中的函数,并使用 `__stdcall` 关键字来指定函数调用约定。例如:
```c++
__declspec(dllexport) int __stdcall MyFunction(int arg1, int arg2)
{
// 函数实现
}
```
这样,在编译 DLL 时,就会将 MyFunction 函数导出为 DLL 中的一个函数,并使用 __stdcall 调用约定,以便其他程序正确调用该函数。在其他程序中调用该函数时,需要使用 `__declspec(dllimport)` 关键字来导入 DLL 中的函数,并使用 __stdcall 调用约定。例如:
```c++
__declspec(dllimport) int __stdcall MyFunction(int arg1, int arg2);
int result = MyFunction(1, 2);
```
需要注意的是,在使用 __stdcall 调用约定时,需要确保被调用函数和调用函数的调用约定一致,否则可能会导致程序崩溃或产生异常。
VS STUDIO C# 调用 C++Builder生成的Dll
在VS Studio C#中调用C++Builder生成的DLL,同样可以使用P/Invoke技术。
首先,在C++Builder中定义一个导出函数,例如:
```cpp
extern "C" __declspec(dllexport) int Add(int a, int b)
{
return a + b;
}
```
然后,将该函数编译成DLL库文件(例如MyCppLibrary.dll)。确保在编译时使用stdcall调用约定,以便能够与C#程序进行正确的交互。
接下来,在C#中使用DllImport特性声明该函数,并调用它:
```csharp
using System;
using System.Runtime.InteropServices;
class Program
{
[DllImport("MyCppLibrary.dll")]
public static extern int Add(int a, int b);
static void Main(string[] args)
{
int result = Add(1, 2);
Console.WriteLine(result); // 输出 "3"
}
}
```
在上面的示例中,DllImport特性指定了C++Builder生成的DLL库文件名和函数名。调用Add函数时,C#会自动将参数和返回值转换为正确的类型。
需要注意的是,C++Builder和C#程序都需要使用相同的ABI(Application Binary Interface)。如果C++Builder使用了其他调用约定,需要在DllImport特性中指定 CallingConvention 参数。
阅读全文
相关推荐
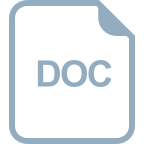
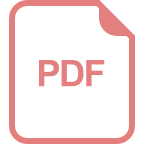
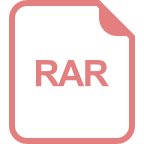
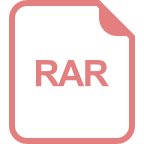
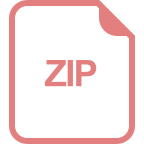
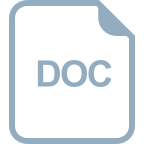
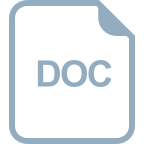
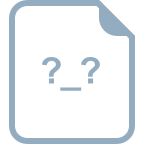
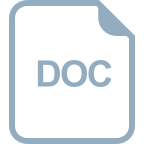
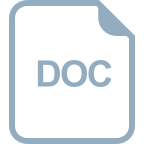
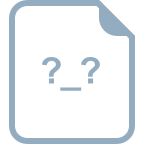
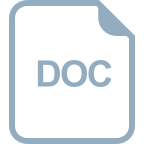
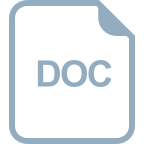
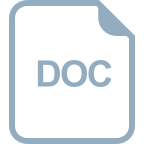
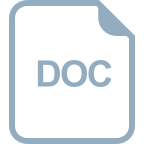
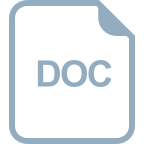

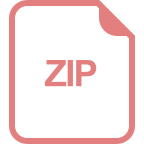