java如何查询一个字符串是否在一个数据库中任意表中存在
时间: 2024-03-27 20:38:27 浏览: 15
在Java中查询一个字符串是否在数据库中任意表中存在,需要进行以下步骤:
1. 首先,建立一个数据库连接,可以使用JDBC连接数据库。
2. 然后,使用SQL语句编写查询语句,查询该字符串在所有表中是否存在。
3. 通过JDBC的Statement或PreparedStatement对象执行查询语句,并获取查询结果。
4. 遍历查询结果,如果存在该字符串,则返回true,否则返回false。
以下是一个简单的示例代码:
```java
import java.sql.*;
public class CheckStringInDB {
public static void main(String[] args) {
Connection con = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 1. 建立数据库连接
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "root";
String password = "password";
con = DriverManager.getConnection(url, user, password);
// 2. 编写查询语句
String query = "SELECT * FROM information_schema.columns WHERE column_name LIKE '%mystring%'";
// 3. 执行查询语句
stmt = con.createStatement();
rs = stmt.executeQuery(query);
// 4. 遍历查询结果
if (rs.next()) {
System.out.println("String exists in database.");
} else {
System.out.println("String does not exist in database.");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 5. 关闭连接和查询结果
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (con != null) con.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
```
这个示例代码是在MySQL数据库中查询一个字符串是否在所有表中存在。需要注意的是,查询语句需要根据具体的数据库类型和表结构进行修改。
相关推荐
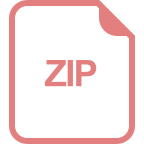
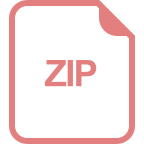
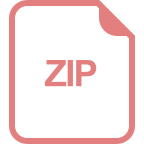














