python 写一个马赛克检测
时间: 2023-07-04 08:17:16 浏览: 177
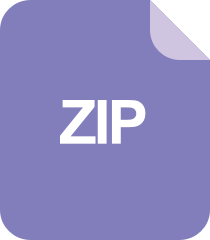
图像马赛克检测程序

好的,下面是一个简单的 Python 马赛克检测实现:
首先,你需要导入 cv2 和 numpy 库:
```python
import cv2
import numpy as np
```
然后,读取待检测的图像:
```python
img = cv2.imread('path/to/image.jpg')
```
接下来,你需要将图像缩放到一个较小的尺寸,以加快处理速度:
```python
scale_percent = 25
width = int(img.shape[1] * scale_percent / 100)
height = int(img.shape[0] * scale_percent / 100)
dim = (width, height)
img = cv2.resize(img, dim, interpolation = cv2.INTER_AREA)
```
然后,你可以使用 cv2.dnn 包中的 cv2.dnn.readNetFromCaffe() 函数加载一个预训练的 Caffe 模型,该模型可以用于检测图像中的人脸:
```python
prototxt_path = 'path/to/deploy.prototxt.txt'
caffemodel_path = 'path/to/res10_300x300_ssd_iter_140000.caffemodel'
net = cv2.dnn.readNetFromCaffe(prototxt_path, caffemodel_path)
```
接下来,你可以使用 cv2.dnn.blobFromImage() 函数将图像转换为一个 blob 对象,该对象可以传递给模型进行预测:
```python
blob = cv2.dnn.blobFromImage(cv2.resize(img, (300, 300)), 1.0,
(300, 300), (104.0, 177.0, 123.0))
```
然后,你可以使用模型进行预测:
```python
net.setInput(blob)
detections = net.forward()
```
最后,你可以遍历检测结果,并将每个检测到的人脸区域用一个马赛克进行覆盖:
```python
for i in range(0, detections.shape[2]):
confidence = detections[0, 0, i, 2]
if confidence > 0.5:
box = detections[0, 0, i, 3:7] * np.array([width, height, width, height])
(startX, startY, endX, endY) = box.astype("int")
roi = img[startY:endY, startX:endX]
roi = cv2.GaussianBlur(roi, (23, 23), 30)
img[startY:endY, startX:endX] = roi
```
这里使用了 cv2.GaussianBlur() 函数对每个人脸区域进行马赛克处理。
完整的代码如下:
```python
import cv2
import numpy as np
img = cv2.imread('path/to/image.jpg')
scale_percent = 25
width = int(img.shape[1] * scale_percent / 100)
height = int(img.shape[0] * scale_percent / 100)
dim = (width, height)
img = cv2.resize(img, dim, interpolation = cv2.INTER_AREA)
prototxt_path = 'path/to/deploy.prototxt.txt'
caffemodel_path = 'path/to/res10_300x300_ssd_iter_140000.caffemodel'
net = cv2.dnn.readNetFromCaffe(prototxt_path, caffemodel_path)
blob = cv2.dnn.blobFromImage(cv2.resize(img, (300, 300)), 1.0,
(300, 300), (104.0, 177.0, 123.0))
net.setInput(blob)
detections = net.forward()
for i in range(0, detections.shape[2]):
confidence = detections[0, 0, i, 2]
if confidence > 0.5:
box = detections[0, 0, i, 3:7] * np.array([width, height, width, height])
(startX, startY, endX, endY) = box.astype("int")
roi = img[startY:endY, startX:endX]
roi = cv2.GaussianBlur(roi, (23, 23), 30)
img[startY:endY, startX:endX] = roi
cv2.imshow('Mosaic Detection', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意,这只是一个简单的马赛克检测实现,它仅检测人脸并对其进行马赛克处理。如果你要检测其他类型的物体或区域,你需要选择适当的模型并进行相应的更改。
阅读全文
相关推荐
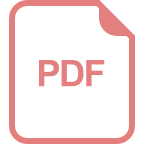
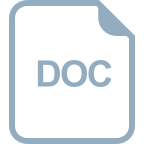
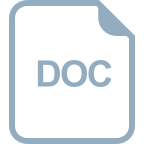




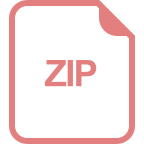
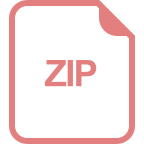
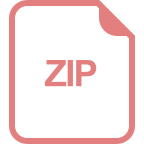
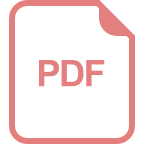
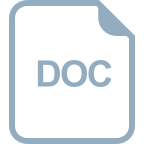



