c语言实现某班级的图书信息管理,图书的基本信息包括书号、书名、作者、单价、数量和简介(50字内)等。源代码
时间: 2023-07-10 09:19:39 浏览: 52
好的,以下是一个简单的 C 语言程序,可以实现图书信息管理系统的基本功能。请注意,这只是一个基本的示例,您可以根据需要进行修改和扩展。
```c
#include <stdio.h>
#include <string.h>
#define MAX_BOOKS 100
#define MAX_DESCRIPTION 50
struct Book {
char number[20];
char name[50];
char author[20];
float price;
int quantity;
char description[MAX_DESCRIPTION];
};
struct Book books[MAX_BOOKS];
int num_books = 0;
void add_book() {
if (num_books == MAX_BOOKS) {
printf("Error: the maximum number of books has been reached.\n");
return;
}
struct Book new_book;
printf("Enter the book number: ");
scanf("%s", new_book.number);
printf("Enter the book name: ");
scanf("%s", new_book.name);
printf("Enter the book author: ");
scanf("%s", new_book.author);
printf("Enter the book price: ");
scanf("%f", &new_book.price);
printf("Enter the book quantity: ");
scanf("%d", &new_book.quantity);
printf("Enter the book description (50 characters or less): ");
scanf("%s", new_book.description);
books[num_books] = new_book;
num_books++;
printf("The book has been added successfully.\n");
}
void search_book() {
char keyword[50];
printf("Enter the keyword to search: ");
scanf("%s", keyword);
int found = 0;
for (int i = 0; i < num_books; i++) {
if (strstr(books[i].name, keyword) != NULL || strstr(books[i].author, keyword) != NULL) {
printf("Book number: %s\n", books[i].number);
printf("Book name: %s\n", books[i].name);
printf("Book author: %s\n", books[i].author);
printf("Book price: %f\n", books[i].price);
printf("Book quantity: %d\n", books[i].quantity);
printf("Book description: %s\n", books[i].description);
printf("\n");
found = 1;
}
}
if (!found) {
printf("No matching books found.\n");
}
}
void list_books() {
if (num_books == 0) {
printf("No books found.\n");
return;
}
printf("Book list:\n");
for (int i = 0; i < num_books; i++) {
printf("Book number: %s\n", books[i].number);
printf("Book name: %s\n", books[i].name);
printf("Book author: %s\n", books[i].author);
printf("Book price: %f\n", books[i].price);
printf("Book quantity: %d\n", books[i].quantity);
printf("Book description: %s\n", books[i].description);
printf("\n");
}
}
int main() {
int choice;
do {
printf("1. Add a book\n");
printf("2. Search for a book\n");
printf("3. List all books\n");
printf("4. Exit\n");
printf("Enter your choice (1-4): ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book();
break;
case 2:
search_book();
break;
case 3:
list_books();
break;
case 4:
printf("Exiting the program.\n");
break;
default:
printf("Invalid choice. Please try again.\n");
break;
}
} while (choice != 4);
return 0;
}
```
这个程序使用结构体来表示每本书的信息。它提供了三个主要功能:添加新书、搜索书籍和列出所有书籍。您可以根据需要添加其他功能,如删除书籍和更新书籍信息。
相关推荐
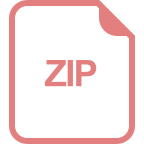














