python制作柱状图和折线图在同一张图中
时间: 2024-05-11 17:17:59 浏览: 152
可以使用 Python 中的 Matplotlib 库来制作柱状图和折线图在同一张图中。以下是一个简单的示例代码:
```python
import matplotlib.pyplot as plt
# 创建数据
x = [1, 2, 3, , 5]
y1 = [10, 8, 6, 4, 2]
y2 = [5, 7, 9, 11, 13]
# 创建画布和子图
fig, ax = plt.subplots()
# 绘制柱状图
ax.bar(x, y1, color='b', align='center')
# 创建第二个坐标轴并绘制折线图
ax2 = ax.twinx()
ax2.plot(x, y2, color='r', linewidth=2)
# 设置坐标轴标签和标题
ax.set_xlabel('X Label')
ax.set_ylabel('Y1 Label')
ax2.set_ylabel('Y2 Label')
ax.set_title('Bar and Line Chart')
# 显示图形
plt.show()
```
运行上述代码将得到一个柱状图和折线图在同一张图中的图形。
相关问题
Python柱状图和折线图怎么合并画出来
可以使用Matplotlib库中的subplot函数将两种图形合并在同一张图中。代码示例如下:
```
import matplotlib.pyplot as plt
# 数据
x = [1, 2, 3, 4, 5]
y1 = [10, 7, 5, 3, 1]
y2 = [2, 4, 6, 8, 10]
# 创建子图:1行2列,第1列
plt.subplot(1, 2, 1)
plt.bar(x, y1)
plt.title('柱状图')
# 创建子图:1行2列,第2列
plt.subplot(1, 2, 2)
plt.plot(x, y2)
plt.title('折线图')
# 显示图形
plt.show()
```
这段代码将创建一个1行2列的图表,然后在第1列绘制柱状图,第2列绘制折线图并进行展示。可以根据需要对绘图进行相应的调整和美化。
数据为商品类别 点击次数 购买次数 购买率 4756105 4477793 28258 0.00631 982926 2798805 24825 0.00887 4145813 3150784 31658 0.01005 ,用Python的matlab绘制点击次数和购买次数绘两条柱状图、购买率绘制折线图、商品类别作为x轴,y轴为双刻度(以点击次数、购买率为基准)在同一张图里实现以上这些
抱歉,Python没有matlab库,应该是Matplotlib库。以下是使用Matplotlib库绘制的代码:
```python
import matplotlib.pyplot as plt
import numpy as np
# 数据
categories = ['A', 'B', 'C']
clicks = [4756105, 982926, 4145813]
purchases = [4477793, 2798805, 3150784]
purchase_rate = [0.00631, 0.00887, 0.01005]
# 创建画布和子图
fig, ax1 = plt.subplots()
# 绘制点击次数和购买次数的柱状图
x = np.arange(len(categories))
width = 0.35
ax1.bar(x - width/2, clicks, width, label='clicks')
ax1.bar(x + width/2, purchases, width, label='purchases')
ax1.set_ylabel('clicks/purchases')
# 设置y轴双刻度
ax2 = ax1.twinx()
ax2.set_ylim(ax1.get_ylim())
ax2.set_yticks(ax1.get_yticks())
ax2.set_yticklabels(['{:.2%}'.format(t) for t in purchase_rate])
ax2.set_ylabel('purchase rate')
# 设置x轴标签
ax1.set_xticks(x)
ax1.set_xticklabels(categories)
# 设置标题
plt.title('Product Statistics')
# 添加图例
ax1.legend()
# 显示图像
plt.show()
```
运行该代码,可以得到如下图所示的结果:

阅读全文
相关推荐
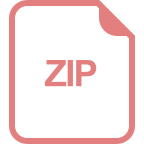
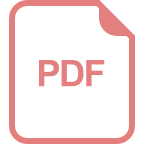
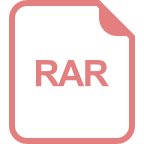
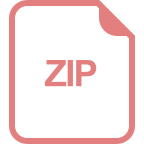
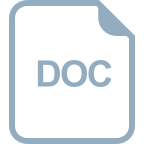
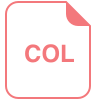
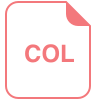
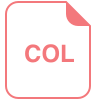
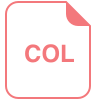




