在Android Studio中使用Java实现一个扫雷游戏
时间: 2024-05-18 20:13:23 浏览: 154
好的,下面是一个简单的扫雷游戏的 Java 实现,仅供参考:
首先,在 Android Studio 中创建一个新的项目,并创建一个名为 Minesweeper 的 Activity。
然后,创建一个名为 Cell 的类,表示每个扫雷格子的状态,包括是否被揭示、是否有地雷、以及周围有多少个地雷等信息。代码如下:
```
public class Cell {
private boolean isRevealed;
private boolean hasBomb;
private int bombsAround;
public Cell() {
isRevealed = false;
hasBomb = false;
bombsAround = 0;
}
public boolean isRevealed() {
return isRevealed;
}
public void setRevealed(boolean revealed) {
isRevealed = revealed;
}
public boolean hasBomb() {
return hasBomb;
}
public void setHasBomb(boolean hasBomb) {
this.hasBomb = hasBomb;
}
public int getBombsAround() {
return bombsAround;
}
public void setBombsAround(int bombsAround) {
this.bombsAround = bombsAround;
}
}
```
接下来,在 Minesweeper Activity 中创建一个二维数组来表示整个扫雷游戏的状态,并初始化每个格子为一个 Cell 对象。代码如下:
```
public class Minesweeper extends AppCompatActivity {
private final int ROWS = 10;
private final int COLS = 10;
private final int BOMBS = 10;
private Cell[][] cells = new Cell[ROWS][COLS];
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_minesweeper);
initializeCells();
placeBombs();
calculateBombsAround();
}
private void initializeCells() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cells[i][j] = new Cell();
}
}
}
private void placeBombs() {
int bombsPlaced = 0;
while (bombsPlaced < BOMBS) {
int row = (int) (Math.random() * ROWS);
int col = (int) (Math.random() * COLS);
if (!cells[row][col].hasBomb()) {
cells[row][col].setHasBomb(true);
bombsPlaced++;
}
}
}
private void calculateBombsAround() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
int bombsAround = 0;
for (int row = i - 1; row <= i + 1; row++) {
for (int col = j - 1; col <= j + 1; col++) {
if (row >= 0 && row < ROWS && col >= 0 && col < COLS) {
if (cells[row][col].hasBomb()) {
bombsAround++;
}
}
}
}
cells[i][j].setBombsAround(bombsAround);
}
}
}
}
```
在 initializeCells() 方法中,我们创建了一个 ROWS * COLS 大小的 cells 数组,并将每个格子初始化为一个 Cell 对象。
在 placeBombs() 方法中,我们随机放置了 BOMBS 个地雷。为了避免重复放置地雷,我们使用了一个 while 循环来不断尝试放置地雷,直到放置了足够的地雷为止。
在 calculateBombsAround() 方法中,我们计算了每个格子周围有多少个地雷,并将结果存储在对应的 Cell 对象中。
最后,我们需要在布局文件中创建一个 GridView 来显示扫雷游戏的界面,并使用一个自定义的适配器来将每个格子的状态显示出来。代码如下:
```
public class CellAdapter extends BaseAdapter {
private Context context;
private Cell[][] cells;
public CellAdapter(Context context, Cell[][] cells) {
this.context = context;
this.cells = cells;
}
@Override
public int getCount() {
return cells.length * cells[0].length;
}
@Override
public Object getItem(int position) {
int row = position / cells[0].length;
int col = position % cells[0].length;
return cells[row][col];
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
int row = position / cells[0].length;
int col = position % cells[0].length;
TextView textView = new TextView(context);
textView.setLayoutParams(new GridView.LayoutParams(40, 40));
textView.setGravity(Gravity.CENTER);
if (cells[row][col].isRevealed()) {
if (cells[row][col].hasBomb()) {
textView.setBackgroundColor(Color.RED);
} else {
textView.setBackgroundColor(Color.WHITE);
textView.setText(String.valueOf(cells[row][col].getBombsAround()));
}
} else {
textView.setBackgroundColor(Color.GRAY);
}
return textView;
}
}
```
在 Minesweeper Activity 中,我们使用 GridView 和 CellAdapter 来显示扫雷游戏的界面。代码如下:
```
public class Minesweeper extends AppCompatActivity {
private final int ROWS = 10;
private final int COLS = 10;
private final int BOMBS = 10;
private Cell[][] cells = new Cell[ROWS][COLS];
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_minesweeper);
initializeCells();
placeBombs();
calculateBombsAround();
GridView gridView = findViewById(R.id.grid_view);
gridView.setNumColumns(COLS);
gridView.setAdapter(new CellAdapter(this, cells));
}
// ...
}
```
这样,一个简单的扫雷游戏就完成了。当用户点击某个格子时,我们只需要将对应的 Cell 对象的 isRevealed 属性设置为 true,并更新适配器即可。
阅读全文
相关推荐
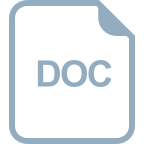
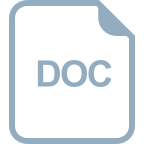
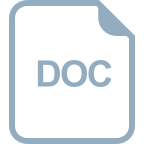


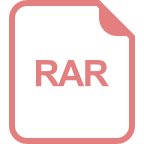
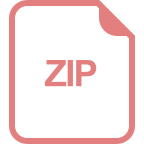
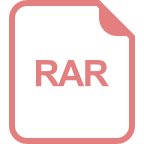
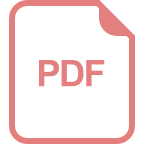
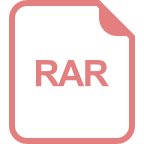
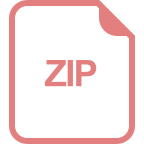
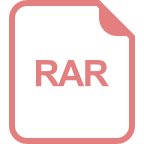
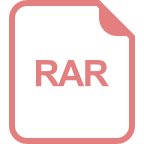





