写一个扫雷游戏,开发软件Android studio,语言Java
时间: 2023-12-25 22:06:38 浏览: 148
以下是一个简单的扫雷游戏示例代码,使用Android Studio开发,使用Java语言编写。
activity_main.xml文件:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/main_layout"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/timeView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_marginTop="8dp"
android:textSize="20sp"
android:text="已用时间:0分0秒"
android:layout_centerHorizontal="true"/>
<GridView
android:id="@+id/gridView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/timeView"
android:numColumns="10"
android:horizontalSpacing="1dp"
android:verticalSpacing="1dp"
android:stretchMode="columnWidth"
android:gravity="center"/>
<LinearLayout
android:id="@+id/button_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_alignParentBottom="true">
<Button
android:id="@+id/startGame"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="开始游戏"
android:layout_weight="1"/>
<Button
android:id="@+id/btn_flippe"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="翻开"
android:layout_weight="1"/>
<Button
android:id="@+id/btn_flag"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="标记"
android:layout_weight="1"/>
</LinearLayout>
</RelativeLayout>
```
MainActivity.java文件:
```java
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.AdapterView;
import android.widget.Button;
import android.widget.GridView;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private static final int MESSAGE_UPDATE_TIME = 1;
private int gameTime = 0;
private boolean gameStarted = false;
private GridView gridView;
private Button startGameButton;
private Button flippeButton;
private Button flagButton;
private TextView timeView;
private MineSweeperAdapter adapter;
private MineSweeperGame game;
private Handler handler = new Handler() {
@Override
public void handleMessage(Message msg) {
if (msg.what == MESSAGE_UPDATE_TIME) {
timeView.setText("已用时间:" + gameTime / 60 + "分" + gameTime % 60 + "秒");
gameTime++;
if (gameStarted) {
handler.sendEmptyMessageDelayed(MESSAGE_UPDATE_TIME, 1000);
}
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
gridView = findViewById(R.id.gridView);
startGameButton = findViewById(R.id.startGame);
flippeButton = findViewById(R.id.btn_flippe);
flagButton = findViewById(R.id.btn_flag);
timeView = findViewById(R.id.timeView);
adapter = new MineSweeperAdapter(this);
game = new MineSweeperGame(10, 10, 10);
gridView.setAdapter(adapter);
gridView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
if (!gameStarted) {
gameStarted = true;
handler.sendEmptyMessage(MESSAGE_UPDATE_TIME);
}
if (game.isGameOver()) {
Toast.makeText(MainActivity.this, "游戏已结束", Toast.LENGTH_SHORT).show();
return;
}
int row = position / game.getColumns();
int col = position % game.getColumns();
if (game.flippe(row, col)) {
adapter.updateView();
if (game.isGameWin()) {
Toast.makeText(MainActivity.this, "游戏胜利", Toast.LENGTH_SHORT).show();
handler.removeMessages(MESSAGE_UPDATE_TIME);
}
} else {
Toast.makeText(MainActivity.this, "游戏失败", Toast.LENGTH_SHORT).show();
handler.removeMessages(MESSAGE_UPDATE_TIME);
}
}
});
startGameButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
game.reset();
adapter.updateView();
gameTime = 0;
gameStarted = false;
timeView.setText("已用时间:0分0秒");
handler.removeMessages(MESSAGE_UPDATE_TIME);
}
});
flippeButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
game.setMode(MineSweeperGame.Mode.FLIPPE);
flippeButton.setBackgroundColor(getResources().getColor(android.R.color.holo_green_light));
flagButton.setBackgroundColor(getResources().getColor(android.R.color.white));
}
});
flagButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
game.setMode(MineSweeperGame.Mode.FLAG);
flagButton.setBackgroundColor(getResources().getColor(android.R.color.holo_red_light));
flippeButton.setBackgroundColor(getResources().getColor(android.R.color.white));
}
});
}
private class MineSweeperAdapter extends BaseAdapter {
private Context context;
public MineSweeperAdapter(Context context) {
this.context = context;
}
@Override
public int getCount() {
return game.getRows() * game.getColumns();
}
@Override
public Object getItem(int position) {
int row = position / game.getColumns();
int col = position % game.getColumns();
return game.getCell(row, col);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ImageView imageView;
if (convertView == null) {
imageView = new ImageView(context);
imageView.setLayoutParams(new GridView.LayoutParams(GridView.LayoutParams.MATCH_PARENT, 128));
imageView.setScaleType(ImageView.ScaleType.CENTER);
imageView.setPadding(4, 4, 4, 4);
} else {
imageView = (ImageView) convertView;
}
int row = position / game.getColumns();
int col = position % game.getColumns();
int cell = game.getCell(row, col);
if (cell == MineSweeperGame.CELL_UNCOVERED) {
int mineCount = game.getAdjacentMines(row, col);
imageView.setImageResource(mineCount == 0 ? R.drawable.ic_empty : R.drawable.ic_number);
imageView.setBackgroundResource(android.R.color.white);
} else if (cell == MineSweeperGame.CELL_FLAG) {
imageView.setImageResource(R.drawable.ic_flag);
imageView.setBackgroundResource(android.R.color.white);
} else if (cell == MineSweeperGame.CELL_MINE) {
imageView.setImageResource(R.drawable.ic_mine);
imageView.setBackgroundResource(android.R.color.white);
} else {
imageView.setImageResource(R.drawable.ic_covered);
imageView.setBackgroundResource(android.R.color.white);
}
return imageView;
}
public void updateView() {
notifyDataSetChanged();
}
}
}
```
在该示例代码中,我们使用了`GridView`来展示扫雷游戏的棋盘,使用`Handler`和`Message`实现了已用时间的显示和更新,使用`Adapter`和`View`实现了UI界面的更新和显示。通过`reset()`方法重置游戏,通过`isGameOver()`和`isGameWin()`方法实现了游戏结束的判断,通过`flippe()`和`flag()`方法实现了游戏的翻开和标记操作。
需要注意的是,这里的示例代码只是一个简单的扫雷游戏实现,并不完整和健壮,实际开发中还需要考虑更多的细节和异常情况。
阅读全文
相关推荐







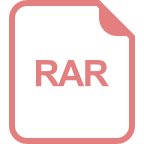
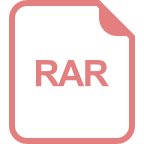

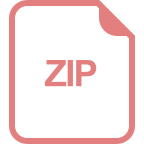







