void read() { vector<string> row; string line; string filename; ifstream in("test5000.csv"); if (in.fail()) { cout << "File not found" <<endl; return ; } while(getline(in, line) && in.good() ) { file_to_string(row, line, ','); //把line里的单元格数字字符提取出来,“,”为单元格分隔符 for(int i=0, leng=row.size(); i<leng; i++){ b.push_back(string_to_float(row[i])); } a.push_back(b); b.clear(); } in.close(); return ; }
时间: 2024-03-30 22:35:27 浏览: 106
这段代码是一个读取CSV文件的函数read(),其主要作用是将CSV文件中的数据读取到内存中的二维数组a中。具体的实现过程是:
1. 打开名为"test5000.csv"的CSV文件,如果打开失败则输出"File not found"并返回。
2. 逐行读取文件内容,每行内容以字符串形式存储在变量line中。
3. 调用函数file_to_string()将line中的单元格数字字符提取出来,并以vector<string>的形式存储在变量row中。
4. 遍历row中的每一个元素,将其转换为float类型并存储在一个一维数组b中。
5. 将一维数组b添加到二维数组a中,并清空一维数组b。
6. 重复步骤2-5,直到文件读取完毕。
7. 关闭文件。
最终,函数read()将读取到的CSV文件数据存储在全局变量a中,供其他函数调用。
相关问题
vector<string>读取数据
vector<string>是C++标准库中的容器,用于存储字符串类型的数据。可以通过以下步骤来读取数据到vector<string>中:
1. 包含头文件:首先需要包含<vector>和<string>头文件。
2. 创建vector<string>对象:使用vector<string>来存储读取到的字符串数据。
3. 打开文件:使用fstream库中的ifstream类打开要读取的文件。
4. 逐行读取数据:使用getline函数从文件中逐行读取数据,并将每行数据存储到vector<string>中。
5. 关闭文件:读取完数据后,关闭文件。
下面是一个示例代码,演示了如何使用vector<string>读取数据:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> data; // 创建vector<string>对象
std::ifstream file("data.txt"); // 打开文件
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) { // 逐行读取数据
data.push_back(line); // 将每行数据存储到vector<string>中
}
file.close(); // 关闭文件
} else {
std::cout << "Failed to open file." << std::endl; }
// 输出读取到的数据
for (const auto& str : data) {
std::cout << str << std::endl;
}
return 0;
}
```
请注意,上述示例代码假设存在名为"data.txt"的文本文件,其中包含要读取的数据,每行一个字符串。
如何对多个vector<string>数据进行文件读写
要对多个`vector<string>`数据进行文件读写,你可以使用循环来处理每个`vector<string>`并进行读取和写入操作。下面是一个示例代码,演示如何对多个`vector<string>`数据进行文件读写:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
void writeDataToFile(const std::vector<std::string>& data, const std::string& filename) {
std::ofstream outputFile(filename);
if (outputFile.is_open()) {
for (const auto& str : data) {
outputFile << str << std::endl;
}
outputFile.close();
std::cout << "数据写入文件 " << filename << " 完成!" << std::endl;
} else {
std::cout << "无法创建输出文件 " << filename << "!" << std::endl;
}
}
void readDataFromFile(std::vector<std::string>& data, const std::string& filename) {
std::ifstream file(filename);
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
data.push_back(line);
}
file.close();
std::cout << "文件 " << filename << " 读取完成!" << std::endl;
} else {
std::cout << "无法打开文件 " << filename << "!" << std::endl;
}
}
int main() {
std::vector<std::vector<std::string>> allData;
// 生成示例数据
std::vector<std::string> data1 = {"Hello", "World"};
std::vector<std::string> data2 = {"C++", "Programming"};
std::vector<std::string> data3 = {"File", "IO"};
// 将数据存储到allData
allData.push_back(data1);
allData.push_back(data2);
allData.push_back(data3);
// 写入数据到文件
for (int i = 0; i < allData.size(); i++) {
std::string filename = "output" + std::to_string(i) + ".txt";
writeDataToFile(allData[i], filename);
}
// 从文件中读取数据
std::vector<std::vector<std::string>> readData;
for (int i = 0; i < allData.size(); i++) {
std::string filename = "output" + std::to_string(i) + ".txt";
std::vector<std::string> tempData;
readDataFromFile(tempData, filename);
readData.push_back(tempData);
}
// 输出读取的数据
for (const auto& data : readData) {
std::cout << "文件内容:" << std::endl;
for (const auto& str : data) {
std::cout << str << std::endl;
}
std::cout << std::endl;
}
return 0;
}
```
上述代码将生成三个`vector<string>`数据,并将它们存储在`allData`中。然后,它使用循环将每个`vector<string>`写入不同的文件中,文件名按照"output0.txt"、"output1.txt"、"output2.txt"的格式命名。
接下来,它再次使用循环从文件中读取数据,并将每个`vector<string>`存储到`readData`中。最后,它输出读取的数据。
请确保在运行代码之前,创建一个新的空文件夹作为输出文件的目录。
阅读全文
相关推荐
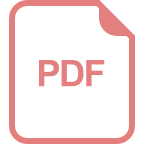
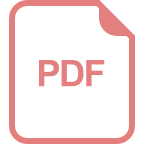
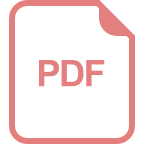













