C++中通过opencv读取txt文件,并将其解析为vector<string>格式
时间: 2023-08-31 14:30:13 浏览: 204
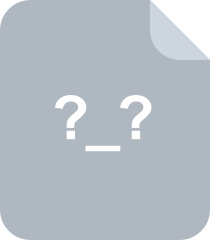
opencv显示出txt文本中的内容

### 回答1:
在 C++ 中使用 OpenCV 读取 txt 文件并将其解析为 vector<string> 格式可以使用以下代码:
```
#include <opencv2/opencv.hpp>
#include <vector>
#include <string>
using namespace std;
int main() {
string filename = "example.txt";
vector<string> data;
ifstream file(filename);
if(file.is_open()) {
string line;
while(getline(file, line)) {
data.push_back(line);
}
file.close();
}
return 0;
}
```
在这段代码中,我们首先声明了一个文件名变量,一个 vector 变量 data 和一个 ifstream 变量 file。然后我们使用 ifstream 类的构造函数打开文件,并使用 is_open() 函数判断文件是否正常打开。如果文件打开成功,我们使用 getline() 函数逐行读取文件的内容并将每行的内容插入到 vector 变量 data 中。最后,我们关闭文件。
### 回答2:
在C中通过OpenCV读取txt文件,并将其解析为vector<string>格式的步骤如下:
1. 导入必要的头文件:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <opencv2/opencv.hpp>
```
2. 创建一个函数来读取txt文件并解析为vector<string>格式:
```c++
std::vector<std::string> readTXTFile(const std::string& filename) {
std::vector<std::string> lines;
std::ifstream file(filename);
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
lines.push_back(line);
}
file.close();
} else {
std::cout << "Error opening file: " << filename << std::endl;
}
return lines;
}
```
3. 使用OpenCV中的 imread 函数读取txt文件:
```c++
std::string filename = "data.txt"; // txt文件名
cv::Mat image = cv::imread(filename, cv::IMREAD_GRAYSCALE);
```
4. 将读取的图像数据解析为vector<string>格式:
```c++
std::vector<std::string> lines;
for (int i = 0; i < image.rows; i++) {
std::string line;
for (int j = 0; j < image.cols; j++) {
int pixel_value = static_cast<int>(image.at<uchar>(i, j));
line += std::to_string(pixel_value) + " ";
}
lines.push_back(line);
}
```
5. 打印解析后的数据:
```c++
for (const auto& line : lines) {
std::cout << line << std::endl;
}
```
以上是通过OpenCV在C中读取txt文件并将其解析为vector<string>格式的步骤。
### 回答3:
在C++中利用OpenCV读取txt文件并解析为vector<string>格式可以通过以下步骤实现:
1. 首先,需要包含OpenCV的头文件和iostream头文件:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
```
2. 接下来,创建一个函数来读取txt文件并解析为vector<string>格式,函数原型如下:
```c++
std::vector<std::string> readTxtFile(const std::string& filename);
```
3. 在函数中,首先定义一个存储解析后字符串的vector<string>,例如:
```c++
std::vector<std::string> strVector;
```
4. 使用OpenCV的FileStorage类来读取txt文件:
```c++
cv::FileStorage fs(filename, cv::FileStorage::READ);
if (!fs.isOpened()) {
std::cerr << "Failed to open txt file." << std::endl;
return strVector;
}
```
5. 利用OpenCV的FileNode和FileNodeIterator对文件进行解析:
```c++
cv::FileNode rootNode = fs["data"];
if (rootNode.empty()) {
std::cerr << "Empty data node." << std::endl;
return strVector;
}
cv::FileNodeIterator it = rootNode.begin();
cv::FileNodeIterator it_end = rootNode.end();
for (; it != it_end; ++it) {
std::string str = (std::string)*it;
strVector.push_back(str);
}
```
6. 最后,返回解析后的字符串向量:
```c++
return strVector;
```
以下是完整的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
std::vector<std::string> readTxtFile(const std::string& filename) {
std::vector<std::string> strVector;
cv::FileStorage fs(filename, cv::FileStorage::READ);
if (!fs.isOpened()) {
std::cerr << "Failed to open txt file." << std::endl;
return strVector;
}
cv::FileNode rootNode = fs["data"];
if (rootNode.empty()) {
std::cerr << "Empty data node." << std::endl;
return strVector;
}
cv::FileNodeIterator it = rootNode.begin();
cv::FileNodeIterator it_end = rootNode.end();
for (; it != it_end; ++it) {
std::string str = (std::string)*it;
strVector.push_back(str);
}
return strVector;
}
int main() {
std::vector<std::string> strVector = readTxtFile("data.txt");
for (const auto& str : strVector) {
std::cout << str << std::endl;
}
return 0;
}
```
这样,就可以通过OpenCV读取txt文件并解析为vector<string>格式。
阅读全文
相关推荐

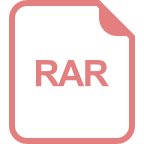













