c/c++利用opencv读取文件夹中的视频,并将视频的rgb信息按照视频名保存在另一个文件夹下
时间: 2024-02-09 11:11:38 浏览: 15
可以使用以下代码实现:
```C++
#include <opencv2/opencv.hpp>
#include <iostream>
#include <string>
#include <vector>
#include <fstream>
using namespace cv;
using namespace std;
int main()
{
string folder_path = "path/to/video/folder/"; // 视频文件夹路径,注意最后要加上斜杠
string output_folder = "path/to/output/folder/"; // RGB信息输出文件夹路径,注意最后要加上斜杠
vector<string> video_files; // 保存视频文件名
glob(folder_path + "*.mp4", video_files, false); // 获取视频文件名
for (size_t i = 0; i < video_files.size(); i++)
{
string video_path = video_files[i];
string video_name = video_path.substr(video_path.find_last_of('/') + 1); // 获取视频文件名
cout << "Processing video " << video_name << endl;
VideoCapture cap(video_path); // 打开视频
if (!cap.isOpened())
{
cerr << "Unable to open video file: " << video_name << endl;
continue;
}
int frame_count = static_cast<int>(cap.get(CAP_PROP_FRAME_COUNT)); // 获取视频总帧数
int fps = static_cast<int>(cap.get(CAP_PROP_FPS)); // 获取视频帧率
int width = static_cast<int>(cap.get(CAP_PROP_FRAME_WIDTH)); // 获取视频宽度
int height = static_cast<int>(cap.get(CAP_PROP_FRAME_HEIGHT)); // 获取视频高度
Mat frame;
ofstream outfile(output_folder + video_name + ".txt"); // 创建保存RGB信息的文件
for (int j = 0; j < frame_count; j++)
{
cap.read(frame); // 读取一帧
if (frame.empty()) // 如果读取失败,退出循环
{
cerr << "Empty frame detected in video file: " << video_name << endl;
break;
}
// 将每个像素的RGB信息写入文件,格式为 R,G,B\n
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++)
{
Vec3b pixel = frame.at<Vec3b>(y, x);
outfile << static_cast<int>(pixel[2]) << "," << static_cast<int>(pixel[1]) << "," << static_cast<int>(pixel[0]) << "\n";
}
}
}
outfile.close(); // 关闭文件
}
return 0;
}
```
这段代码会遍历指定文件夹下所有的MP4格式视频文件,依次读取每个视频,并将每一帧的RGB信息按照指定的格式保存到输出文件夹下以视频名命名的文本文件中。要使用该程序,需将代码中的路径替换为实际路径。
相关推荐
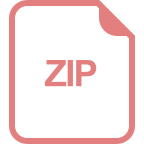
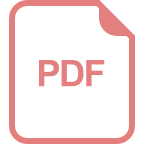
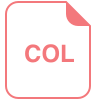
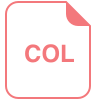
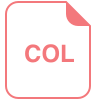











