使用c/c++利用opnecv批量读取文件夹中的图片,并依次保存其rgb信息在本地中
时间: 2024-02-09 09:08:24 浏览: 176
好的,现在我开始对你的问题进行回答。
首先,需要安装OpenCV库。然后,使用以下代码可以读取文件夹中的所有图片,并将其RGB信息保存到本地文件中:
```c
#include <opencv2/opencv.hpp>
#include <iostream>
#include <fstream>
#include <string>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
string folder = "path/to/folder"; // 替换为你的文件夹路径
string output = "output.txt"; // 替换为你要输出的文件路径
ofstream out(output);
if (!out.is_open()) {
cerr << "无法打开输出文件 " << output << endl;
return -1;
}
vector<String> filenames;
glob(folder, filenames);
int count = 0;
for (auto &filename : filenames)
{
Mat img = imread(filename, IMREAD_COLOR);
if (img.empty()) {
cerr << "无法读取文件 " << filename << endl;
continue;
}
// 保存RGB信息到输出文件中
for (int i = 0; i < img.rows; i++) {
for (int j = 0; j < img.cols; j++) {
Vec3b pixel = img.at<Vec3b>(i, j);
out << (int)pixel[0] << ' ' << (int)pixel[1] << ' ' << (int)pixel[2] << endl;
}
}
count++;
}
out.close();
cout << "读取并保存了 " << count << " 张图片的 RGB 信息到 " << output << " 中" << endl;
return 0;
}
```
这段代码会遍历指定的文件夹,读取所有的图片,并将它们的RGB信息保存到输出文件中。你可以将路径和文件名替换为你想要的路径和文件名。
阅读全文
相关推荐







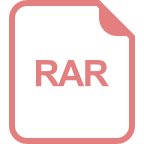
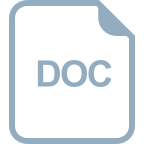





