c/c++利用opencv读取文件夹中的图片,并将图片的rgb信息按照每个图片名保存在另一个文件夹下
时间: 2023-09-10 22:05:52 浏览: 236
你好,您可以使用C++语言中的OpenCV库来实现此功能。以下是一个基本的示例代码:
```
#include <opencv2/opencv.hpp>
#include <iostream>
#include <string>
using namespace cv;
using namespace std;
int main()
{
string folderPath = "path/to/folder"; // 图片文件夹的路径
string savePath = "path/to/save/folder"; // 存储文件夹的路径
vector<String> files;
glob(folderPath, files); // 获取所有文件的文件名
for (size_t i = 0; i < files.size(); ++i)
{
Mat img = imread(files[i]); // 读取图片文件
if (!img.empty())
{
string fileName = files[i].substr(files[i].find_last_of("\\/") + 1); // 获取文件名,需要根据操作系统做出相应修改
string saveFileName = savePath + "\\" + fileName.substr(0, fileName.find(".")) + ".txt"; // 生成每个图片名对应的 txt 文件名
ofstream outFile(saveFileName); // 创建 txt 文件
// 输出每个像素点的 RGB 信息到 txt 文件
for (int y = 0; y < img.rows; ++y)
{
for (int x = 0; x < img.cols; ++x)
{
Vec3b pixel = img.at<Vec3b>(y, x); // 获取当前像素点的 RGB 值
outFile << int(pixel[0]) << " " << int(pixel[1]) << " " << int(pixel[2]) << endl;
}
}
outFile.close(); // 关闭文件流
}
}
return 0;
}
```
上述代码可以遍历指定文件夹中的所有图片,将每张图片的 RGB 信息按照图片名保存在另一个文件夹下。您可以根据实际需求进行相应修改。希望能对您有所帮助。
阅读全文
相关推荐








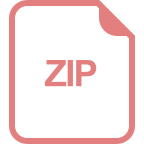





