c/c++利用opencv读取文件夹中的视频,并将视频的每一帧的rgb信息按照每个视频名保存在另一个文件夹下
时间: 2024-02-09 08:11:10 浏览: 76
您可以使用OpenCV中的VideoCapture函数来读取视频,并使用imwrite函数将每个帧的RGB信息保存到另一个文件夹中。下面是一些示例代码来帮助您开始实现:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
#include <string>
int main()
{
std::string folder_path = "/path/to/folder/containing/videos/"; // path to folder containing videos
std::string save_path = "/path/to/save/RGB/frames/"; // path to save RGB frames
cv::VideoCapture cap;
cv::Mat frame;
std::vector<cv::Mat> channels;
std::string video_name;
int count = 1;
// loop through all videos in folder
for (const auto& file : std::filesystem::directory_iterator(folder_path))
{
// open video file
cap.open(file.path().string());
// check if video opened successfully
if (!cap.isOpened())
{
std::cerr << "Error opening video file: " << file.path().string() << std::endl;
continue;
}
// get video name without extension
video_name = file.path().stem().string();
// create folder to save RGB frames
std::filesystem::create_directory(save_path + video_name);
// loop through all frames in video
while (cap.read(frame))
{
// split frame into RGB channels
cv::split(frame, channels);
// save each channel to separate file with format "video_name-frame_index-channel_name.jpg"
cv::imwrite(save_path + video_name + "/" + video_name + "-" + std::to_string(count) + "-red.jpg", channels[2]);
cv::imwrite(save_path + video_name + "/" + video_name + "-" + std::to_string(count) + "-green.jpg", channels[1]);
cv::imwrite(save_path + video_name + "/" + video_name + "-" + std::to_string(count) + "-blue.jpg", channels[0]);
count++;
}
// reset frame count for next video
count = 1;
// release video file
cap.release();
}
return 0;
}
```
这段代码将打开指定文件夹中的所有视频文件,并将每个帧分离为RGB通道。然后,它将把每个通道保存为其自己的文件,其中文件名使用视频名称、帧索引和通道名称构造。请注意,您需要将文件夹路径和保存路径更改为适合您的输入和输出文件夹路径。
阅读全文
相关推荐












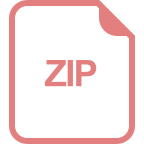

