OpenCV C++提取文字
时间: 2023-08-24 19:08:27 浏览: 177
OpenCV提取文字通常需要进行以下步骤:
1. 读取图像:使用OpenCV中的imread函数读取图像。
2. 图像预处理:对图像进行预处理,如灰度化、二值化、去噪等操作,以便于文字的识别。
3. 文字定位:使用轮廓检测等方法定位图像中的文字区域。
4. 文字识别:对文字区域进行识别,可以使用OCR库或者深度学习模型进行识别。
5. 文字后处理:对识别结果进行后处理,如去除错误识别、合并断字等。
下面是一个使用OpenCV和Tesseract OCR库进行文字识别的示例代码:
```cpp
#include <opencv2/opencv.hpp>
#include <tesseract/baseapi.h>
using namespace cv;
int main()
{
// 读取图像
Mat img = imread("test.jpg");
// 图像预处理
cvtColor(img, img, COLOR_BGR2GRAY);
threshold(img, img, 0, 255, THRESH_BINARY_INV | THRESH_OTSU);
medianBlur(img, img, 3);
// 文字定位
std::vector<std::vector<Point>> contours;
findContours(img.clone(), contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
std::vector<Rect> rects;
for (const auto& contour : contours)
{
Rect rect = boundingRect(contour);
if (rect.width > rect.height * 3 && rect.width > 30 && rect.height > 30)
{
rects.push_back(rect);
}
}
// 文字识别
tesseract::TessBaseAPI ocr;
ocr.Init(NULL, "eng", tesseract::OEM_LSTM_ONLY);
ocr.SetPageSegMode(tesseract::PSM_SINGLE_BLOCK);
for (const auto& rect : rects)
{
Mat roi = img(rect);
ocr.SetImage(roi.data, roi.cols, roi.rows, 1, roi.cols);
ocr.Recognize(0);
std::string text = ocr.GetUTF8Text();
std::cout << text << std::endl;
}
return 0;
}
```
上述代码将读取名为test.jpg的图像,进行灰度化、二值化和中值滤波等预处理操作,然后使用轮廓检测定位文字区域,最后使用Tesseract OCR库进行文字识别。
阅读全文
相关推荐

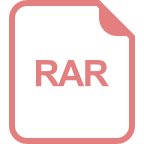
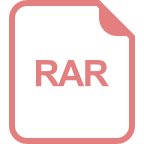
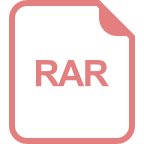
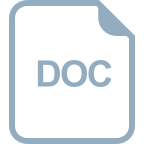
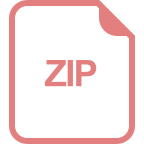
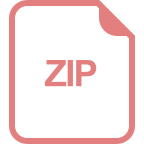
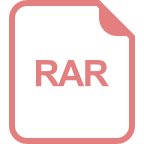
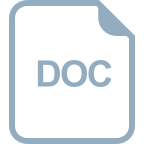
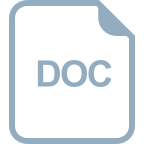

