将析构函数改成虚析构函数
时间: 2024-05-10 16:14:58 浏览: 8
当一个类中有虚函数时,如果该类的对象被删除,只会调用该类的析构函数,不会调用任何派生类的析构函数。这就会导致派生类中的资源没有被释放,从而出现内存泄漏。
为了解决这个问题,需要将析构函数改成虚析构函数。虚析构函数是指在基类中将析构函数声明为虚函数,这样当一个对象的指针被删除时,会调用该对象所属类的析构函数,从而保证所有资源被正确释放。
示例代码如下:
```cpp
class Base {
public:
virtual ~Base() {
// 基类析构函数
}
};
class Derived : public Base {
public:
~Derived() override {
// 派生类析构函数
}
};
```
在上面的代码中,`Base` 类的析构函数被声明为虚析构函数,而 `Derived` 类的析构函数使用了 `override` 关键字覆盖了基类的虚析构函数。这样,当一个 `Derived` 类的对象被删除时,会先调用 `Derived` 类的析构函数,再调用 `Base` 类的析构函数,从而保证资源被正确释放。
相关问题
c++中析构函数虚函数
在C++中,析构函数可以被定义为虚函数。虚析构函数是一个在基类中定义的虚函数,它在派生类中被重写。当我们使用基类指针来删除派生类对象时,如果析构函数不是虚函数,那么只会调用基类的析构函数,而不会调用派生类的析构函数,这样就会导致派生类中的资源没有被释放。而如果析构函数是虚函数,那么在删除派生类对象时,会先调用派生类的析构函数,再调用基类的析构函数,这样就可以保证派生类中的资源被正确释放。
下面是一个示例代码,其中演示了基类指针操作派生类对象时,基类析构函数是虚函数的情况:
```
在C++中,析构函数
将析构函数设置为虚函数报错
析构函数可以声明为虚函数,而构造函数不能声明为虚函数的原因是因为在对象的构造过程中,对象的类型是逐渐确定的,而在析构过程中,对象的类型已经确定。引用\[1\]中提到,构造函数在对象创建时被调用,此时对象的内存已经分配,但是vtable还没有初始化。因此,在构造函数中调用虚函数会导致无法找到正确的vtable,从而引发错误。而在析构函数中,对象的类型已经确定,vtable已经初始化,因此可以安全地调用虚函数。引用\[2\]中提到,如果析构函数不声明为虚函数,那么通过基类指针销毁派生类对象时,只会调用基类的析构函数,而不会调用派生类的析构函数,这会导致对象没有正确地被销毁。因此,为了确保正确的对象销毁,析构函数应该声明为虚函数。引用\[3\]中提到,如果在基类中声明了纯虚函数,并在基类的析构函数中调用该纯虚函数,编译器会报错,因为在析构函数中调用纯虚函数是没有意义的。所以,将析构函数设置为虚函数是合理且必要的。
#### 引用[.reference_title]
- *1* *2* *3* [构造函数可以调用虚函数吗,析构函数可以调用虚函数吗(1)](https://blog.csdn.net/qq_55125921/article/details/126076938)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
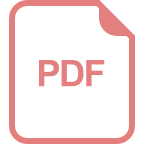
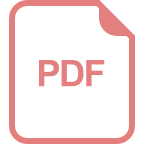
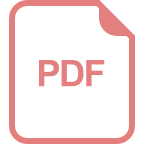












