将c语言线性表的代码改成类的形式并封装
时间: 2023-06-14 20:06:03 浏览: 135
以下是一个简单的 C++ 类实现线性表的例子,包括封装的私有成员变量和公有成员函数,以及一些基本的线性表操作:
```cpp
#include <iostream>
class LinearList {
private:
int* data; // 线性表数据
int length; // 线性表长度
int capacity; // 线性表容量
public:
// 构造函数,初始化线性表
LinearList(int cap): length(0), capacity(cap) {
data = new int[capacity];
}
// 析构函数,释放内存
~LinearList() {
delete[] data;
}
// 插入元素
bool insert(int index, int value) {
if (index < 0 || index > length) {
return false;
}
if (length >= capacity) {
return false;
}
for (int i = length; i > index; i--) {
data[i] = data[i-1];
}
data[index] = value;
length++;
return true;
}
// 删除元素
bool remove(int index) {
if (index < 0 || index >= length) {
return false;
}
for (int i = index; i < length-1; i++) {
data[i] = data[i+1];
}
length--;
return true;
}
// 获取元素
bool get(int index, int& value) {
if (index < 0 || index >= length) {
return false;
}
value = data[index];
return true;
}
// 设置元素
bool set(int index, int value) {
if (index < 0 || index >= length) {
return false;
}
data[index] = value;
return true;
}
// 获取线性表长度
int size() {
return length;
}
// 判断线性表是否为空
bool empty() {
return length == 0;
}
// 清空线性表
void clear() {
length = 0;
}
// 打印线性表
void print() {
std::cout << "[";
for (int i = 0; i < length; i++) {
std::cout << data[i];
if (i < length-1) {
std::cout << ", ";
}
}
std::cout << "]" << std::endl;
}
};
```
这个类的实现中,私有成员变量 `data`、`length` 和 `capacity` 用于存储线性表的数据、长度和容量,分别表示线性表的数组、长度和最大容量。公有成员函数包括插入、删除、获取、设置、获取长度、判断是否为空、清空和打印等操作,这些操作对外暴露,可以被其他代码调用。通过这个类的封装,我们可以更方便地使用线性表,避免了直接使用数组时可能出现的错误和不便利。
阅读全文
相关推荐
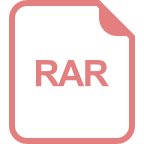
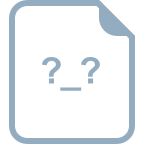
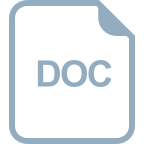
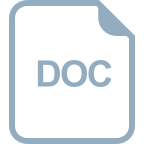
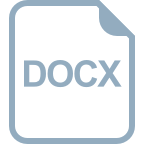
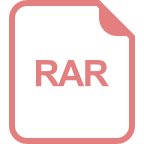
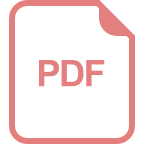
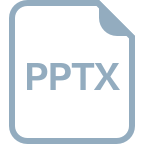
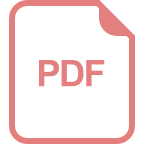
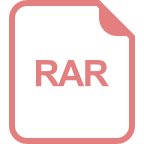
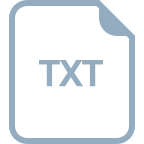
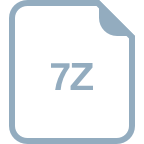
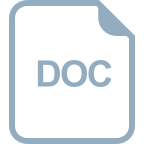
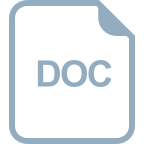