用c语言将线性表的增删改查用代码实现
时间: 2024-02-05 07:13:53 浏览: 25
这里提供一个基于数组实现的线性表的增删改查的C语言代码示例:
```c
#include <stdio.h>
#define MAX_SIZE 100 // 线性表的最大长度
int list[MAX_SIZE]; // 线性表数组
int length = 0; // 线性表当前长度
/* 在线性表末尾插入元素 */
void insert(int value)
{
if (length >= MAX_SIZE) {
printf("Error: Linear list is full!\n");
return;
}
list[length++] = value;
}
/* 删除线性表中指定位置的元素 */
void delete(int index)
{
if (index < 0 || index >= length) {
printf("Error: Index out of range!\n");
return;
}
for (int i = index; i < length - 1; i++) {
list[i] = list[i+1];
}
length--;
}
/* 修改线性表中指定位置的元素 */
void modify(int index, int value)
{
if (index < 0 || index >= length) {
printf("Error: Index out of range!\n");
return;
}
list[index] = value;
}
/* 查找线性表中第一个值为value的元素的位置,如果没有则返回-1 */
int search(int value)
{
for (int i = 0; i < length; i++) {
if (list[i] == value) {
return i;
}
}
return -1;
}
/* 输出线性表中的所有元素 */
void print()
{
printf("Linear list: ");
for (int i = 0; i < length; i++) {
printf("%d ", list[i]);
}
printf("\n");
}
int main()
{
insert(1);
insert(2);
insert(3);
print(); // Linear list: 1 2 3
delete(1);
print(); // Linear list: 1 3
modify(1, 4);
print(); // Linear list: 1 4
int index = search(4);
if (index != -1) {
printf("Index of 4: %d\n", index); // Index of 4: 1
} else {
printf("4 not found!\n");
}
return 0;
}
```
以上是一个简单的线性表增删改查的C语言代码示例,仅供参考。
相关推荐
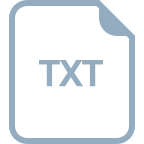
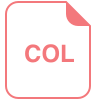
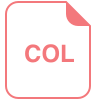
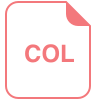
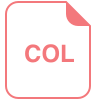
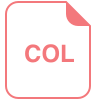









