QThread内函数有哪些,各有什么作用
时间: 2023-04-11 18:01:03 浏览: 53
作为C知道,我可以回答你的问题。QThread内函数包括:
1. run()函数:这是QThread中最重要的函数,它定义了线程的执行内容。
2. start()函数:这个函数用于启动线程,它会自动调用run()函数。
3. wait()函数:这个函数用于等待线程执行完毕。
4. quit()函数:这个函数用于结束线程的执行。
5. sleep()函数:这个函数用于让线程暂停一段时间。
6. currentThreadId()函数:这个函数用于获取当前线程的ID。
7. isRunning()函数:这个函数用于判断线程是否正在运行。
这些函数各有不同的作用,可以根据需要来使用。
相关问题
QThread 的quit函数和wait函数有什么区别
QThread 的 quit() 函数和 wait() 函数都是用于控制线程的函数,但是它们的作用不同。
quit() 函数是用于停止线程的执行,通常在线程的 run() 函数中使用。当调用 quit() 函数时,QThread 会向线程发送一个停止信号,线程会尽快退出 run() 函数的执行。但是,这种停止方式不是立即生效的,需要等到线程执行完当前的代码块后才会停止。
wait() 函数是用于等待线程执行完毕的函数,通常在主线程中使用。当调用 wait() 函数时,主线程会一直等待,直到线程执行完 run() 函数的所有操作后才会返回。如果线程在执行过程中被强制停止,wait() 函数也会立即返回。
简而言之,quit() 函数是用于停止线程的执行,而 wait() 函数是用于等待线程执行完毕。
c++ Qt 中QThread 的quit函数和wait函数有什么区别
C Qt 中 QThread 的 quit() 函数和 wait() 函数与 Python Qt 中的用法类似,也都是用于控制线程的函数,但是它们的作用也是不同的。
quit() 函数是用于停止线程的执行,通常在线程的 run() 函数中使用。当调用 quit() 函数时,QThread 会向线程发送一个停止信号,线程会尽快退出 run() 函数的执行。但是,这种停止方式不是立即生效的,需要等到线程执行完当前的代码块后才会停止。
wait() 函数是用于等待线程执行完毕的函数,通常在主线程中使用。当调用 wait() 函数时,主线程会一直等待,直到线程执行完 run() 函数的所有操作后才会返回。如果线程在执行过程中被强制停止,wait() 函数也会立即返回。
简而言之,quit() 函数是用于停止线程的执行,而 wait() 函数是用于等待线程执行完毕。
以下是一个 C++ Qt 中的简单例子:
```cpp
#include <QCoreApplication>
#include <QThread>
#include <QDebug>
class MyThread : public QThread
{
public:
void run() override
{
while (true) {
qDebug() << "Hello from MyThread";
}
}
};
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
MyThread thread;
thread.start();
thread.quit();
thread.wait();
return app.exec();
}
```
在上述代码中,我们定义了一个 MyThread 类,其中 run() 函数中有一个无限循环,每次循环都会输出一句话。在主线程中,我们创建了 MyThread 类型的对象 thread,并调用了它的 start() 函数来启动线程。然后,我们调用了 thread.quit() 函数来停止线程的执行,然后调用了 thread.wait() 函数等待线程执行完毕。当线程执行完毕后,主线程会继续执行,从而退出应用程序。
相关推荐
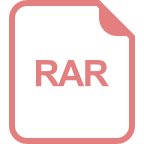
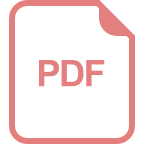
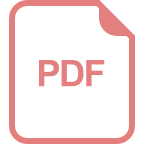












