最小生成树构造-prim算法
时间: 2023-12-04 18:41:49 浏览: 83
以下是使用Prim算法构造最小生成树的步骤:
1. 选择一个起始点作为根节点,将其加入到生成树中。
2. 找到与生成树相邻的所有边中权重最小的那条边,将其连接的节点加入到生成树中。
3. 重复步骤2,直到所有节点都被加入到生成树中。
下面是使用Prim算法构造最小生成树的Python代码示例:
```python
import heapq
def prim(graph, start):
visited = set([start])
edges = [
(cost, start, to)
for to, cost in graph[start].items()
]
heapq.heapify(edges)
mst_cost = 0
while edges:
cost, frm, to = heapq.heappop(edges)
if to not in visited:
visited.add(to)
mst_cost += cost
for to_next, cost in graph[to].items():
if to_next not in visited:
heapq.heappush(edges, (cost, to, to_next))
if len(visited) == len(graph):
return mst_cost
else:
return "impossible"
```
其中,`graph`是一个字典,表示图的邻接表,`start`是起始节点。函数返回最小生成树的树边权重之和,如果最小生成树不存在则返回`impossible`。
相关推荐
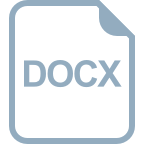
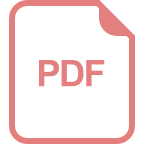
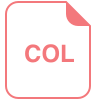
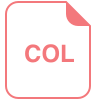
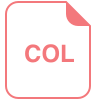
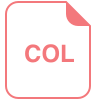
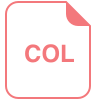









