C语言实现哈希表的结构体,创建,插入,查找
时间: 2024-10-19 20:11:14 浏览: 40
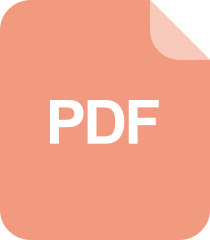
C语言基于哈希表实现通讯录

在C语言中,我们可以使用数组结合下标操作来模拟哈希表的基本功能。一个简单的哈希表可以包含一个固定大小的数组和一个函数(通常称为哈希函数),用于计算键值对的索引位置。
```c
typedef struct {
int size; // 哈希表的大小
int capacity; // 容量,一般大于size
char* data; // 数组存储数据,实际是一个指向void指针的指针,因为不同类型的数据可以存储
} HashTable;
// 创建哈希表
HashTable* createHashTable(int size) {
HashTable* table = (HashTable*)malloc(sizeof(HashTable));
if (!table) return NULL;
table->size = size;
table->capacity = size * 2; // 为了防止冲突,通常初始化容量为两倍
table->data = malloc(table->capacity * sizeof(void*)); // 初始化数组
if (!table->data) {
free(table);
return NULL;
}
for (int i = 0; i < table->capacity; i++) {
table->data[i] = NULL; // 初始化每个元素为NULL
}
return table;
}
// 插入元素
void insert(HashTable* table, const void* key, void* value) {
int index = hashFunction(key, table->size); // 根据key计算索引
while (table->data[index] != NULL) { // 如果该位置已有元素,寻找下一个空位
index = (index + 1) % table->capacity;
}
table->data[index] = value; // 将value存储在找到的位置
}
// 查找元素
void* search(HashTable* table, const void* key) {
int index = hashFunction(key, table->size);
while (table->data[index] != NULL) {
if (table->data[index] == key) return table->data[index]; // 如果找到相同的key,返回其对应的value
index = (index + 1) % table->capacity;
}
return NULL; // 如果没找到,返回NULL
}
// 哈希函数示例(这里仅作演示,实际使用需根据键的特性选择合适的哈希函数)
unsigned int hashFunction(const void* key, int size) {
unsigned int hash = (unsigned int)key;
return hash % size;
}
```
阅读全文
相关推荐
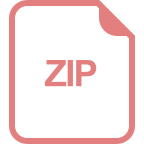
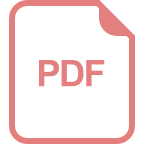
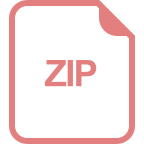
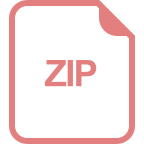
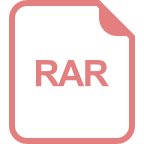
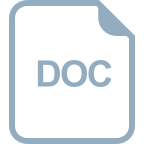
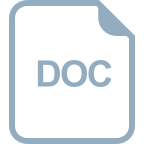
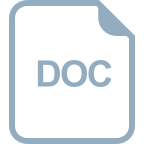
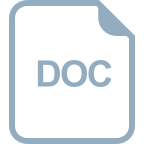
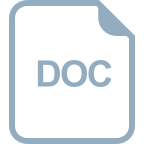







