在pandas中,哪个方法用于dataframe对象中选择某些列,A:set_columns() B:set_rows() C:loc() D:iloc()
时间: 2024-02-06 08:04:22 浏览: 112
在 pandas 中,选择 DataFrame 对象中的某些列,可以使用 `loc[]` 或 `iloc[]` 方法,因此正确的答案是 C 或 D。
`loc[]` 方法用于基于标签(如列名)选择数据,而 `iloc[]` 方法用于基于整数位置(如列索引)选择数据。两种方法都可以用来选择行和列。例如:
```python
# 使用 loc[] 方法选择 'col1' 和 'col2' 两列
df.loc[:, ['col1', 'col2']]
# 使用 iloc[] 方法选择第 1 和第 3 列
df.iloc[:, [0, 2]]
```
需要注意的是,`set_columns()` 和 `set_rows()` 方法在 pandas 中并不存在,因此选项 A 和 B 都是错误的。
相关问题
在python中pandas提供了一些与read相关的函数,可以读取文件中的数据并形成dataframe,再利用dataframe进行数据分析、预处理、以及行、列操作等。请将代码块中下划线替换成相应代码,使用read函数读取“固定资产信息.XLS”,并根据提示完成数据清洗。# 引入pandas #显示所有列 pd.set_option('display.max_columns', None) #显示所有行 pd.set_option('display.max_rows', None) # 读取‘固定资产信息.xls’表 # 输出内容显示前五行 #按列进行判断是否有空值,并输出 #在原DataFrame上将缺失值使用数值类型‘0’填充 #将结果打印输出
```python
# 引入pandas
import pandas as pd
# 显示所有列
pd.set_option('display.max_columns', None)
# 显示所有行
pd.set_option('display.max_rows', None)
# 读取‘固定资产信息.xls’表
df = pd.read_excel('固定资产信息.xls')
# 输出内容显示前五行
print(df.head())
# 按列进行判断是否有空值,并输出
print(df.isnull().any())
# 在原DataFrame上将缺失值使用数值类型‘0’填充
df = df.fillna(0)
# 将结果打印输出
print(df)
```
注:以上代码假设“固定资产信息.xls”文件与该Python文件在同一目录下。如果文件路径不同,需要相应修改文件路径。
帮我看一下这段代码哪里有问题,怎么修改?import pandas as pd import numpy as np pd.set_option('display.max_columns', None)#所有列 pd.set_option('display.max_rows', None)#所有行 data=pd.read_excel('半监督数据.xlsx') datas = pd.DataFrame(data)dataset=datas(labeled_size=0.3,test_size=0.1,stratified=False,shuffle=True,random_state=0, default_transforms=True)
这段代码存在几个问题:
1. 缺少导入 `sklearn.model_selection.train_test_split` 的语句,这个函数用于划分数据集。
2. `datas(labeled_size=0.3,test_size=0.1,stratified=False,shuffle=True,random_state=0,default_transforms=True)` 中的 `labeled_size` 和 `test_size` 参数应该使用 `train_size` 和 `test_size` 代替。
3. `datas` 是一个 DataFrame 对象,应该使用 `train_test_split` 函数对其进行划分,而不是将其作为参数传递给 `dataset`。
修改后的代码如下:
```
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
pd.set_option('display.max_columns', None) # 所有列
pd.set_option('display.max_rows', None) # 所有行
data = pd.read_excel('半监督数据.xlsx')
X = data.drop(columns=['label']) # 特征矩阵
y = data['label'] # 标签列
# 划分数据集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.1, stratify=None, shuffle=True, random_state=0)
# 划分带标签数据集
labeled_size = 0.3
n_labeled = int(labeled_size * len(X_train))
indices = np.arange(len(X_train))
unlabeled_indices = np.delete(indices, y_train.index[:n_labeled])
X_unlabeled = X_train.iloc[unlabeled_indices]
y_unlabeled = y_train.iloc[unlabeled_indices]
X_labeled = X_train.iloc[y_train.index[:n_labeled]]
y_labeled = y_train.iloc[y_train.index[:n_labeled]]
```
这里将数据集划分为带标签数据集和无标签数据集,只对带标签数据集进行训练。如果需要同时使用带标签数据集和无标签数据集进行训练,可以使用半监督学习的算法,例如标签传播算法和自训练算法。
阅读全文
相关推荐
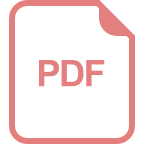
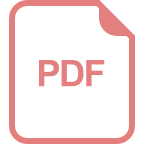








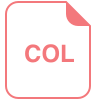




