bayer RAW24 线性插值转彩 c++
时间: 2024-01-31 09:10:27 浏览: 115
以下是使用C++进行Bayer RAW24线性插值转彩的示例代码:
```cpp
#include <iostream>
#include <vector>
// 线性插值函数
int linearInterpolation(int a, int b, int c, int d) {
return (a + b + c + d) / 4;
}
// Bayer RAW24线性插值转彩函数
void bayerToRGB(std::vector<unsigned char>& bayerData, int width, int height, std::vector<unsigned char>& rgbData) {
int rgbWidth = width * 3;
int rgbHeight = height * 3;
rgbData.resize(rgbWidth * rgbHeight);
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int bayerIndex = y * width + x;
int rgbIndex = (y * 3) * rgbWidth + (x * 3);
// 获取周围像素的索引
int leftIndex = y * width + (x - 1);
int rightIndex = y * width + (x + 1);
int topIndex = (y - 1) * width + x;
int bottomIndex = (y + 1) * width + x;
// 获取周围像素的值
int leftPixel = (x > 0) ? bayerData[leftIndex] : 0;
int rightPixel = (x < width - 1) ? bayerData[rightIndex] : 0;
int topPixel = (y > 0) ? bayerData[topIndex] : 0;
int bottomPixel = (y < height - 1) ? bayerData[bottomIndex] : 0;
// 进行线性插值
int red = linearInterpolation(leftPixel, rightPixel, topPixel, bottomPixel);
int green = bayerData[bayerIndex];
int blue = linearInterpolation(topPixel, bottomPixel, leftPixel, rightPixel);
// 将RGB值写入输出数据
rgbData[rgbIndex] = red;
rgbData[rgbIndex + 1] = green;
rgbData[rgbIndex + 2] = blue;
}
}
}
int main() {
// 假设有一个Bayer RAW24数据
std::vector<unsigned char> bayerData = { /* Bayer RAW24数据 */ };
int width = 640;
int height = 480;
// 调用Bayer RAW24线性插值转彩函数
std::vector<unsigned char> rgbData;
bayerToRGB(bayerData, width, height, rgbData);
// 输出转换后的RGB数据
for (int i = 0; i < rgbData.size(); i += 3) {
std::cout << "R: " << (int)rgbData[i] << ", G: " << (int)rgbData[i + 1] << ", B: " << (int)rgbData[i + 2] << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
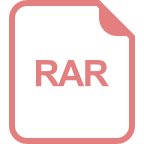
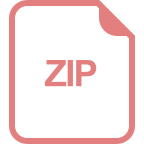

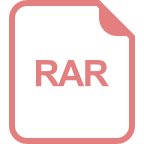
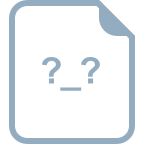
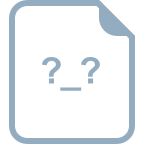
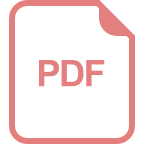
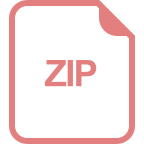
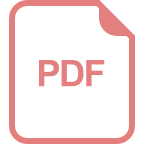
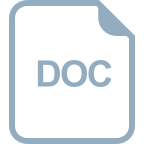
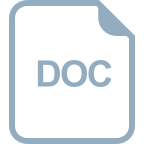





