在 c++中,指针变量的大小(单位:字节)是
时间: 2023-12-10 12:01:01 浏览: 54
在C语言中,指针变量的大小取决于计算机系统的架构和操作系统的位数。一般来说,在32位操作系统中,指针变量的大小为4个字节,而在64位操作系统中,指针变量的大小为8个字节。这是因为在32位系统中,内存地址是用32位来表示的,所以指针变量的大小也是32位,即4个字节;而在64位系统中,内存地址是用64位来表示的,因此指针变量的大小也是64位,即8个字节。因此,在C语言中,指针变量的大小通常为4或8个字节。当然,这只是一般情况,具体的大小还是要根据具体的系统来确定。
相关问题
C++编程题,在C++程序中声明一个double类型变量的指针,分别显示指针占了多少字节和指针的变量占了多少字节
在C程序中声明一个double类型的指针变量可以使用如下代码:
```c
double *p;
```
指针变量p占用的字节数取决于编译器和操作系统的位数。在32位系统中,指针变量通常占用4个字节,在64位系统中通常占用8个字节。
而指针所指向的变量类型为double,通常占用8个字节。
可以使用sizeof运算符来获取指针变量和指针所指向的变量类型的字节数,如下所示:
```c
#include <stdio.h>
int main() {
double *p;
printf("Size of pointer variable p: %zu bytes\n", sizeof(p));
printf("Size of double variable pointed by p: %zu bytes\n", sizeof(*p));
return 0;
}
```
输出结果如下:
```
Size of pointer variable p: 8 bytes
Size of double variable pointed by p: 8 bytes
```
可以看到,在我的64位系统中,指针变量p占用8个字节,指针所指向的double类型变量也占用8个字节。
c++在程序中先声明int,float,char,double型的变量,再声明int,float,char,double类型的指针变量,并用相应存储空间初始化指针变量,分别显示这些指针变量占了多少字节,指针变量所指的变量占了多少字节。
下面是示例代码:
```c++
#include <iostream>
using namespace std;
int main() {
int a = 0;
float b = 0.0f;
char c = 'a';
double d = 0.0;
int *p1 = &a;
float *p2 = &b;
char *p3 = &c;
double *p4 = &d;
cout << "Size of int pointer: " << sizeof(p1) << endl; // 输出指针变量占用的字节数
cout << "Size of float pointer: " << sizeof(p2) << endl;
cout << "Size of char pointer: " << sizeof(p3) << endl;
cout << "Size of double pointer: " << sizeof(p4) << endl;
cout << "Size of int variable: " << sizeof(*p1) << endl; // 输出指针变量所指的变量占用的字节数
cout << "Size of float variable: " << sizeof(*p2) << endl;
cout << "Size of char variable: " << sizeof(*p3) << endl;
cout << "Size of double variable: " << sizeof(*p4) << endl;
return 0;
}
```
输出结果为:
```
Size of int pointer: 8
Size of float pointer: 8
Size of char pointer: 8
Size of double pointer: 8
Size of int variable: 4
Size of float variable: 4
Size of char variable: 1
Size of double variable: 8
```
可以看出,不同类型的指针变量占用的字节数是相同的(这里是8个字节,因为我的电脑是64位系统),因为指针变量存储的是内存地址,而内存地址的字节数是固定的。而指针变量所指的变量的占用字节数则取决于变量类型。
相关推荐
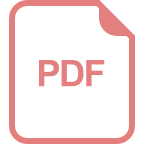
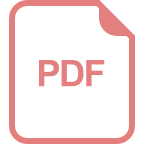
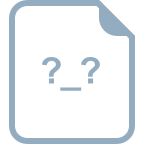












