javaweb购物车代码的实现
时间: 2023-10-26 20:19:51 浏览: 53
实现购物车功能,需要以下几个步骤:
1. 创建购物车类,用于存储商品信息及购物车操作。
```java
public class ShoppingCart {
private List<Product> productList = new ArrayList<Product>();
//添加商品到购物车
public void addProduct(Product product) {
productList.add(product);
}
//删除商品
public void removeProduct(Product product) {
productList.remove(product);
}
//获取购物车中商品列表
public List<Product> getProductList() {
return productList;
}
//计算购物车中商品总价
public double getTotalPrice() {
double totalPrice = 0;
for (Product product : productList) {
totalPrice += product.getPrice();
}
return totalPrice;
}
//清空购物车
public void clearCart() {
productList.clear();
}
}
```
2. 创建商品类,包含商品的基本信息。
```java
public class Product {
private String name; //商品名称
private double price; //商品价格
public Product(String name, double price) {
this.name = name;
this.price = price;
}
//获取商品名称
public String getName() {
return name;
}
//获取商品价格
public double getPrice() {
return price;
}
}
```
3. 在网页中实现添加商品到购物车、删除商品、查看购物车等功能。
```jsp
<%@ page import="java.util.*" %>
<%@ page import="com.example.ShoppingCart" %>
<%@ page import="com.example.Product" %>
<%
//创建购物车对象,并将其存储到session中
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
if (cart == null) {
cart = new ShoppingCart();
session.setAttribute("cart", cart);
}
//获取商品列表,并显示在网页上
List<Product> productList = new ArrayList<Product>();
productList.add(new Product("商品1", 100.0));
productList.add(new Product("商品2", 200.0));
productList.add(new Product("商品3", 300.0));
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>购物车</title>
</head>
<body>
<h1>商品列表</h1>
<table>
<% for (Product product : productList) { %>
<tr>
<td><%= product.getName() %></td>
<td><%= product.getPrice() %>元</td>
<td>
<form action="AddToCartServlet" method="post">
<input type="hidden" name="name" value="<%= product.getName() %>">
<input type="hidden" name="price" value="<%= product.getPrice() %>">
<input type="submit" value="加入购物车">
</form>
</td>
</tr>
<% } %>
</table>
<h1>购物车</h1>
<table>
<% for (Product product : cart.getProductList()) { %>
<tr>
<td><%= product.getName() %></td>
<td><%= product.getPrice() %>元</td>
<td>
<form action="RemoveFromCartServlet" method="post">
<input type="hidden" name="name" value="<%= product.getName() %>">
<input type="hidden" name="price" value="<%= product.getPrice() %>">
<input type="submit" value="删除">
</form>
</td>
</tr>
<% } %>
<tr>
<td>总价:</td>
<td><%= cart.getTotalPrice() %>元</td>
<td>
<form action="ClearCartServlet" method="post">
<input type="submit" value="清空购物车">
</form>
</td>
</tr>
</table>
</body>
</html>
```
4. 创建Servlet实现添加商品、删除商品、清空购物车等操作。
```java
public class AddToCartServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//获取商品信息
String name = request.getParameter("name");
double price = Double.parseDouble(request.getParameter("price"));
Product product = new Product(name, price);
//将商品添加到购物车
ShoppingCart cart = (ShoppingCart) request.getSession().getAttribute("cart");
cart.addProduct(product);
//重定向到购物车页面
response.sendRedirect("cart.jsp");
}
}
public class RemoveFromCartServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//获取商品信息
String name = request.getParameter("name");
double price = Double.parseDouble(request.getParameter("price"));
Product product = new Product(name, price);
//将商品从购物车中删除
ShoppingCart cart = (ShoppingCart) request.getSession().getAttribute("cart");
cart.removeProduct(product);
//重定向到购物车页面
response.sendRedirect("cart.jsp");
}
}
public class ClearCartServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//清空购物车
ShoppingCart cart = (ShoppingCart) request.getSession().getAttribute("cart");
cart.clearCart();
//重定向到购物车页面
response.sendRedirect("cart.jsp");
}
}
```
以上就是基本的购物车功能实现。当然,还可以根据实际需求进行扩展,如加入数量、结算等功能。
相关推荐
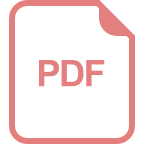














