用Android实现商品详情页。 要求: 1. 点击某一个商品进入本商品的详情页,详情页数据包括商品图片、商品名称、商品价格、该商品的详细介绍。 2. 商品详情页底部包含一个“购买”按钮,点击购买后,跳转到购物车页面。 3. 购物车页面可实现商品数量的增减、商品的删除以及总价格计算功能。给出详细代码,1.adapter要在新建类中,2.使用listview
时间: 2024-02-16 11:04:19 浏览: 20
好的,以下是实现商品详情页的Android代码:
1. 商品详情页布局文件 detail_layout.xml
```
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/img_product"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:scaleType="centerCrop" />
<TextView
android:id="@+id/tv_product_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp"
android:textSize="18sp" />
<TextView
android:id="@+id/tv_product_price"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp"
android:textSize="18sp" />
<TextView
android:id="@+id/tv_product_desc"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp"
android:textSize="16sp" />
<Button
android:id="@+id/btn_buy"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="购买" />
</LinearLayout>
```
2. 商品详情页Activity文件 DetailActivity.java
```
public class DetailActivity extends AppCompatActivity {
private ImageView imgProduct;
private TextView tvProductName, tvProductPrice, tvProductDesc;
private Button btnBuy;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.detail_layout);
imgProduct = findViewById(R.id.img_product);
tvProductName = findViewById(R.id.tv_product_name);
tvProductPrice = findViewById(R.id.tv_product_price);
tvProductDesc = findViewById(R.id.tv_product_desc);
btnBuy = findViewById(R.id.btn_buy);
// 获取商品信息
Intent intent = getIntent();
String productName = intent.getStringExtra("productName");
String productPrice = intent.getStringExtra("productPrice");
String productDesc = intent.getStringExtra("productDesc");
int productImage = intent.getIntExtra("productImage", 0);
// 设置商品信息
imgProduct.setImageResource(productImage);
tvProductName.setText(productName);
tvProductPrice.setText(productPrice);
tvProductDesc.setText(productDesc);
// 设置购买按钮点击事件
btnBuy.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(DetailActivity.this, CartActivity.class);
startActivity(intent);
}
});
}
}
```
3. 购物车页面布局文件 cart_layout.xml
```
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ListView
android:id="@+id/list_cart"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"/>
<TextView
android:id="@+id/tv_total_price"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp"
android:textSize="18sp" />
<Button
android:id="@+id/btn_checkout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="结算" />
</LinearLayout>
```
4. 购物车页面Adapter文件 CartAdapter.java
```
public class CartAdapter extends BaseAdapter {
private List<Product> productList;
private Context context;
public CartAdapter(List<Product> productList, Context context) {
this.productList = productList;
this.context = context;
}
@Override
public int getCount() {
return productList.size();
}
@Override
public Object getItem(int position) {
return productList.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder viewHolder;
if (convertView == null) {
convertView = LayoutInflater.from(context).inflate(R.layout.cart_item, null);
viewHolder = new ViewHolder();
viewHolder.imgProduct = convertView.findViewById(R.id.img_product);
viewHolder.tvProductName = convertView.findViewById(R.id.tv_product_name);
viewHolder.tvProductPrice = convertView.findViewById(R.id.tv_product_price);
viewHolder.btnDecrease = convertView.findViewById(R.id.btn_decrease);
viewHolder.tvProductCount = convertView.findViewById(R.id.tv_product_count);
viewHolder.btnIncrease = convertView.findViewById(R.id.btn_increase);
viewHolder.btnDelete = convertView.findViewById(R.id.btn_delete);
convertView.setTag(viewHolder);
} else {
viewHolder = (ViewHolder) convertView.getTag();
}
Product product = productList.get(position);
viewHolder.imgProduct.setImageResource(product.getProductImage());
viewHolder.tvProductName.setText(product.getProductName());
viewHolder.tvProductPrice.setText(product.getProductPrice());
viewHolder.tvProductCount.setText(String.valueOf(product.getProductCount()));
viewHolder.btnDecrease.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int count = product.getProductCount();
if (count > 1) {
count--;
product.setProductCount(count);
notifyDataSetChanged();
}
}
});
viewHolder.btnIncrease.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int count = product.getProductCount();
count++;
product.setProductCount(count);
notifyDataSetChanged();
}
});
viewHolder.btnDelete.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
productList.remove(position);
notifyDataSetChanged();
}
});
return convertView;
}
class ViewHolder {
ImageView imgProduct;
TextView tvProductName, tvProductPrice, tvProductCount;
Button btnDecrease, btnIncrease, btnDelete;
}
}
```
5. 购物车页面Activity文件 CartActivity.java
```
public class CartActivity extends AppCompatActivity {
private ListView listCart;
private TextView tvTotalPrice;
private Button btnCheckout;
private List<Product> productList = new ArrayList<>();
private CartAdapter cartAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.cart_layout);
listCart = findViewById(R.id.list_cart);
tvTotalPrice = findViewById(R.id.tv_total_price);
btnCheckout = findViewById(R.id.btn_checkout);
// 模拟购物车数据
productList.add(new Product("商品1", "100元", R.drawable.product1, 1));
productList.add(new Product("商品2", "200元", R.drawable.product2, 1));
productList.add(new Product("商品3", "300元", R.drawable.product3, 1));
cartAdapter = new CartAdapter(productList, this);
listCart.setAdapter(cartAdapter);
// 计算总价
double totalPrice = 0;
for (Product product : productList) {
totalPrice += Double.parseDouble(product.getProductPrice().replace("元", "")) * product.getProductCount();
}
tvTotalPrice.setText("总价:" + totalPrice + "元");
// 设置结算按钮点击事件
btnCheckout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(CartActivity.this, "结算成功", Toast.LENGTH_SHORT).show();
}
});
}
}
```
其中,商品实体类Product.java:
```
public class Product {
private String productName;
private String productPrice;
private int productImage;
private int productCount;
public Product(String productName, String productPrice, int productImage, int productCount) {
this.productName = productName;
this.productPrice = productPrice;
this.productImage = productImage;
this.productCount = productCount;
}
public String getProductName() {
return productName;
}
public void setProductName(String productName) {
this.productName = productName;
}
public String getProductPrice() {
return productPrice;
}
public void setProductPrice(String productPrice) {
this.productPrice = productPrice;
}
public int getProductImage() {
return productImage;
}
public void setProductImage(int productImage) {
this.productImage = productImage;
}
public int getProductCount() {
return productCount;
}
public void setProductCount(int productCount) {
this.productCount = productCount;
}
}
```
以上就是实现商品详情页及购物车功能的Android代码,希望能对你有所帮助。
相关推荐
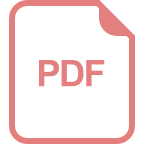
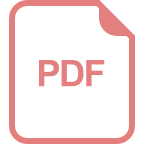











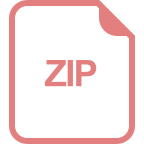
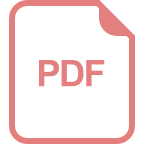