实现生产者和消费者的线程 的C语言代码
时间: 2023-10-20 07:07:41 浏览: 78
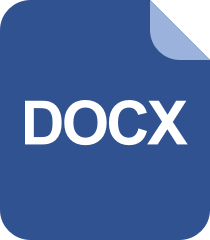
生产者消费者的c++代码实现

下面是一个简单的生产者和消费者线程的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int count = 0;
int in = 0, out = 0;
pthread_mutex_t mutex;
pthread_cond_t full, empty;
void *producer(void *arg)
{
while (1) {
pthread_mutex_lock(&mutex);
if (count == BUFFER_SIZE) {
pthread_cond_wait(&full, &mutex);
}
buffer[in] = rand() % 100;
printf("Produced: %d\n",buffer[in]);
in = (in + 1) % BUFFER_SIZE;
count++;
pthread_mutex_unlock(&mutex);
pthread_cond_signal(&empty);
}
pthread_exit(NULL);
}
void *consumer(void *arg)
{
while (1) {
pthread_mutex_lock(&mutex);
if (count == 0) {
pthread_cond_wait(&empty, &mutex);
}
int item = buffer[out];
printf("Consumed: %d\n", item);
out = (out + 1) % BUFFER_SIZE;
count--;
pthread_mutex_unlock(&mutex);
pthread_cond_signal(&full);
}
pthread_exit(NULL);
}
int main(int argc, char *argv[])
{
pthread_t p, c;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&full, NULL);
pthread_cond_init(&empty, NULL);
pthread_create(&p, NULL, producer, NULL);
pthread_create(&c, NULL, consumer, NULL);
pthread_join(p, NULL);
pthread_join(c, NULL);
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&full);
pthread_cond_destroy(&empty);
return 0;
}
```
这是一个基本的生产者和消费者模型的实现,使用互斥锁和条件变量来保护缓冲区。在这个实现中,生产者向缓冲区中添加元素,消费者从缓冲区中取出元素。当缓冲区已满时,生产者等待其余的消费者线程取走一个元素后再添加一个元素。当缓冲区为空时,消费者等待其余的生产者线程添加一个元素后再取出一个元素。
阅读全文
相关推荐
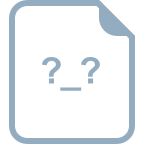
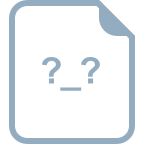

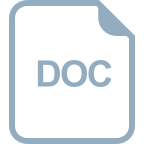
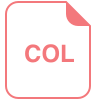












