1. 编写程序,计算某学生奖学金的等级,以三门课程分数为评奖依据。奖学金评奖标准 如下: 符合下列条件之一者获一等奖: (1)平均分大于 95 分者; (2)有两门分数是 100 分,且第三门课程分数不低于 80 分者。 符合下列条件之一者获二等奖: (1)平均分大于 90 分者; (2)有一门分数是 100 分,且其他课程分数不低于 75 分者。 符合下列条件者获三等奖: 各门功课分数不低于 70 分。 符合条件者就高不就低,只能获得高的那一项奖学金。要求显示获奖的等级。
时间: 2024-02-05 15:11:14 浏览: 161
以下是Python代码实现:
```python
score1 = int(input("请输入第一门课程的分数:"))
score2 = int(input("请输入第二门课程的分数:"))
score3 = int(input("请输入第三门课程的分数:"))
average_score = (score1 + score2 + score3) / 3 # 计算平均分
if average_score > 95 or (score1 == 100 and score2 == 100 and score3 >= 80):
print("恭喜你获得一等奖学金!")
elif average_score > 90 or (score1 == 100 and score2 >= 75 and score3 >= 75) or (score2 == 100 and score1 >= 75 and score3 >= 75) or (score3 == 100 and score1 >= 75 and score2 >= 75):
print("恭喜你获得二等奖学金!")
elif score1 >= 70 and score2 >= 70 and score3 >= 70:
print("恭喜你获得三等奖学金!")
else:
print("很遗憾,你没有获得奖学金。")
```
通过用户输入三门课程的分数,计算平均分,并根据奖学金评奖标准判断获奖等级,最后输出获奖等级。
相关问题
假设有三门课程,语文x、数学y、英语z, 三科期末分数均大于90的给一级奖学金,三科均不低于80分且只要有两科高于85的是二等奖学金,三科均不低于80分的是三等奖学金,请编写程序确认同学A能否获得奖学金。用Java程序
为了确认同学A是否能获得奖学金,我们可以创建一个名为`Student`的类,包含三个属性分别代表语文、数学和英语的成绩。然后我们定义三个奖学金方法来检查条件。这里是一个简单的Java程序示例:
```java
public class Student {
private int语文Score;
private int数学Score;
private int英语Score;
public Student(int语文Score, int数学Score, int英语Score) {
this.语文Score = 语文Score;
this.数学Score = 数学Score;
this.EnglishScore = 英语Score;
}
// 判断是否达到一级奖学金条件
public boolean isForFirstScholarship() {
return 语文Score > 90 && 数学Score > 90 && 英语Score > 90;
}
// 判断是否达到二级奖学金条件
public boolean isForSecondScholarship() {
return (语文Score >= 80 && 数学Score >= 80 && 英语Score >= 80)
|| (语文Score > 85 && 数学Score > 85 && 英语Score >= 80)
|| (语文Score > 85 && 数学Score >= 85 && 英语Score > 85);
}
// 判断是否达到三级奖学金条件
public boolean isForThirdScholarship() {
return 语文Score >= 80 && 数学Score >= 80 && 英语Score >= 80;
}
public static void main(String[] args) {
// 创建同学A的成绩实例
Student studentA = new Student(95, 92, 88);
System.out.println("同学A的一级奖学金情况: " + studentA.isForFirstScholarship());
System.out.println("同学A的二级奖学金情况: " + studentA.isForSecondScholarship());
System.out.println("同学A的三级奖学金情况: " + studentA.isForThirdScholarship());
}
}
```
在上述代码中,首先创建了一个学生类,并定义了成绩属性和对应的奖学金判断方法。在`main`函数里,我们假设同学A的语文成绩为95,数学成绩为92,英语成绩为88,然后调用这些方法打印结果,以此判断同学A是否可以获得相应的奖学金。
用python写出下列程序代码1.运用输入输出函数编写程序,能将华氏温度转换成摄氏温度。换算公式:C=(F-32)*5/9,其中C为摄氏温度,F为华氏温度。 2.编写程序,根据输入的长和宽,计算矩形的面积并输出。 3.编写程序,输入三个学生的成绩计算平均分并输出。 4.根据以下叙述写出正确的条件表达式: 有语文(Chinese)、数学(Math)、英语(English)三门课程,均采用百分制,60及以上为及格,90及以上为优秀。 三门课程都及格; 至少一门课程及格; 语文及格且数学或者英语优秀。
1. 华氏温度转摄氏温度的程序代码:
```python
f = float(input("请输入华氏温度:"))
c = (f - 32) * 5 / 9
print("摄氏温度为:", c)
```
2. 计算矩形面积的程序代码:
```python
length = float(input("请输入矩形的长:"))
width = float(input("请输入矩形的宽:"))
area = length * width
print("矩形的面积为:", area)
```
3. 计算学生平均分的程序代码:
```python
score1 = float(input("请输入第一个学生的成绩:"))
score2 = float(input("请输入第二个学生的成绩:"))
score3 = float(input("请输入第三个学生的成绩:"))
avg = (score1 + score2 + score3) / 3
print("三个学生的平均分为:", avg)
```
4. 根据叙述写出条件表达式的程序代码:
```python
# 三门课程都及格
if Chinese >= 60 and Math >= 60 and English >= 60:
print("三门课程都及格")
# 至少一门课程及格
if Chinese >= 60 or Math >= 60 or English >= 60:
print("至少一门课程及格")
# 语文及格且数学或者英语优秀
if Chinese >= 60 and (Math >= 90 or English >= 90):
print("语文及格且数学或者英语优秀")
```
注意,以上代码中的变量 `Chinese`、`Math`、`English` 需要在运行之前先进行定义和赋值。
阅读全文
相关推荐
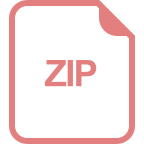
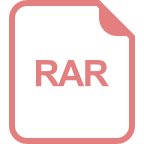













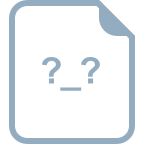