帮我写一个c++的学校选课管理查询系统
时间: 2024-02-18 18:06:22 浏览: 76
好的,这是一个比较大的项目,需要设计数据库、UI界面等,我可以为您提供一个简单的框架,您可以在此基础上进行开发。
首先,我们需要设计一个类来表示学生信息,包括学号、姓名、已选课程等信息,如下所示:
```c++
class Student {
public:
int id; // 学生学号
string name; // 学生姓名
vector<Course> courses; // 已选课程
// 构造函数
Student(int id, string name) {
this->id = id;
this->name = name;
}
// 添加课程
void addCourse(Course course) {
courses.push_back(course);
}
// 删除课程
void removeCourse(int courseId) {
for (int i = 0; i < courses.size(); i++) {
if (courses[i].id == courseId) {
courses.erase(courses.begin() + i);
break;
}
}
}
// 显示已选课程
void showCourses() {
cout << "已选课程:" << endl;
for (int i = 0; i < courses.size(); i++) {
cout << courses[i].name << endl;
}
}
};
```
然后,我们需要设计一个类来表示课程信息,包括课程编号、课程名称、授课教师等信息,如下所示:
```c++
class Course {
public:
int id; // 课程编号
string name; // 课程名称
Teacher teacher; // 授课教师
// 构造函数
Course(int id, string name, Teacher teacher) {
this->id = id;
this->name = name;
this->teacher = teacher;
}
};
```
接下来,我们需要设计一个类来表示教师信息,包括教师编号、姓名等信息,如下所示:
```c++
class Teacher {
public:
int id; // 教师编号
string name; // 教师姓名
// 构造函数
Teacher(int id, string name) {
this->id = id;
this->name = name;
}
};
```
然后,我们需要设计一个类来表示选课系统,包括学生、课程和教师信息的管理,如下所示:
```c++
class CourseSelectionSystem {
public:
vector<Student> students; // 学生信息
vector<Course> courses; // 课程信息
vector<Teacher> teachers; // 教师信息
// 添加学生
void addStudent(Student student) {
students.push_back(student);
}
// 添加课程
void addCourse(Course course) {
courses.push_back(course);
}
// 添加教师
void addTeacher(Teacher teacher) {
teachers.push_back(teacher);
}
// 学生选课
void selectCourse(int studentId, int courseId) {
Student* student = getStudentById(studentId);
Course* course = getCourseById(courseId);
student->addCourse(*course);
}
// 学生退课
void dropCourse(int studentId, int courseId) {
Student* student = getStudentById(studentId);
student->removeCourse(courseId);
}
// 显示学生已选课程
void showStudentCourses(int studentId) {
Student* student = getStudentById(studentId);
student->showCourses();
}
// 获取学生信息
Student* getStudentById(int id) {
for (int i = 0; i < students.size(); i++) {
if (students[i].id == id) {
return &students[i];
}
}
return NULL;
}
// 获取课程信息
Course* getCourseById(int id) {
for (int i = 0; i < courses.size(); i++) {
if (courses[i].id == id) {
return &courses[i];
}
}
return NULL;
}
// 获取教师信息
Teacher* getTeacherById(int id) {
for (int i = 0; i < teachers.size(); i++) {
if (teachers[i].id == id) {
return &teachers[i];
}
}
return NULL;
}
};
```
最后,我们可以在main函数中进行测试,如下所示:
```c++
int main() {
CourseSelectionSystem system;
// 添加学生
Student student1(1, "张三");
Student student2(2, "李四");
system.addStudent(student1);
system.addStudent(student2);
// 添加教师
Teacher teacher1(1, "王老师");
Teacher teacher2(2, "李老师");
system.addTeacher(teacher1);
system.addTeacher(teacher2);
// 添加课程
Course course1(1, "计算机组成原理", teacher1);
Course course2(2, "操作系统", teacher2);
system.addCourse(course1);
system.addCourse(course2);
// 学生选课
system.selectCourse(1, 1);
system.selectCourse(1, 2);
system.selectCourse(2, 1);
// 显示学生已选课程
system.showStudentCourses(1);
system.showStudentCourses(2);
// 学生退课
system.dropCourse(1, 1);
system.showStudentCourses(1);
return 0;
}
```
这样,一个简单的学校选课管理查询系统就完成了。当然,您可以根据自己的需求进行修改和完善。
阅读全文
相关推荐






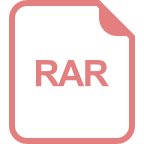


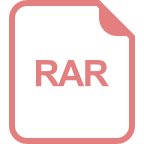

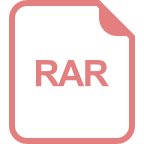
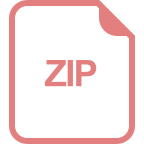



