用python定义一个函数,使字符串的首字母大写
时间: 2024-05-14 11:14:41 浏览: 13
以下是用Python定义一个函数,使字符串的首字母大写的代码:
```python
def capitalize_first_letter(string):
if len(string) == 0:
return string
else:
first_letter = string[0].upper()
rest_of_string = string[1:]
return first_letter + rest_of_string
```
使用`upper()`方法将字符串的第一个字符转换为大写,然后将其与字符串的其余部分拼接起来,最终返回新字符串。
可以这样调用该函数:
```python
string = 'hello world'
new_string = capitalize_first_letter(string)
print(new_string) # 输出:Hello world
```
相关问题
python编写一个函数返回字符串中大写字母个数
好的,以下是一个 Python 函数,可以用来计算字符串中大写字母的数量:
```python
def count_uppercase(s):
count = 0
for c in s:
if c.isupper():
count += 1
return count
```
这个函数接受一个字符串参数 `s`,然后遍历字符串中的每个字符。如果字符是大写字母,那么计数器就加一。最后,函数返回计数器的值,即大写字母的数量。你可以像下面这样调用这个函数:
```python
s = "Hello, World!"
count = count_uppercase(s)
print(count) # 输出:2
```
在这个例子中,字符串 `s` 中有两个大写字母,函数返回值为 2。
可以使用Python的字符串操作函数将输入的字符串首字母大写然后输出。
可以使用Python的字符串操作函数将输入的字符串首字母大写然后输出。比如:
```python
string = input("请输入一个字符串:")
new_str = string.capitalize() # 将字符串首字母大写
print(new_str)
```
或者:
```python
string = input("请输入一个字符串:")
new_str = string[0].upper() + string[1:] # 将字符串第一个字符大写
print(new_str)
```
以上两种方法都可以将输入的字符串转换为首字母大写后输出。第一种方法使用了字符串操作函数capitalize(),第二种方法使用了字符串的切片操作,将第一个字符转换为大写后,将后面的字符与它拼接起来。这样就可以实现将字符串的首字母大写的效果了。
相关推荐
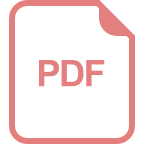
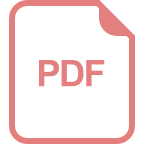
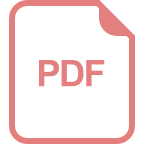
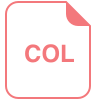
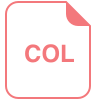
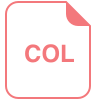
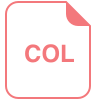
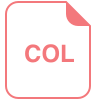







