用python写一段程序,需求1:读取excel中的x与y轴坐标值以及对应的高度。需求2:赋予每个坐标点3种状态,初始状态为0 需求3:读取excel表格中的风速风向数据,根据给定的计算公式计算蔓延因子 需求4:给定初始起火点,即状态从0到1,给定初始速度,乘以蔓延因子得到八个方向上的蔓延速度,乘以时间步长5分钟,得到新的蔓延范围。蔓延时间为1800分钟
时间: 2023-10-24 21:06:44 浏览: 144
以下是一个基本的实现,其中需要注意读取Excel文件需要使用第三方库openpyxl,需要先使用pip安装。
```python
import openpyxl
import math
# 读取Excel文件
wb = openpyxl.load_workbook('data.xlsx')
ws = wb.active
# 读取x、y、高度以及风速风向数据
data = []
for row in ws.iter_rows(min_row=2, max_col=4, values_only=True):
x, y, height, wind = row
data.append((x, y, height, wind))
# 计算蔓延因子
def calculate_spread_factor(wind):
# 根据给定的计算公式计算蔓延因子
return math.sqrt(wind)
# 初始化状态为0
status = [0] * len(data)
# 给每个坐标点3种状态
def set_status(idx, value):
# 如果状态已经为1,则不进行任何修改
if status[idx] == 1:
return
# 如果状态为0,则更新为给定的值
if status[idx] == 0:
status[idx] = value
# 如果状态为2,则需要判断是否需要更新为给定的值
if status[idx] == 2:
if value == 1:
status[idx] = 1
# 给定初始起火点,即状态从0到1
set_status(0, 1)
# 给定初始速度
speed = 10
# 计算蔓延速度
def calculate_spread_speed(idx, spread_factor):
x, y, height, wind = data[idx]
# 计算蔓延速度
spread_speed = speed * spread_factor
return spread_speed
# 计算新的蔓延范围
def calculate_spread_range(idx, spread_speed, time_step):
x, y, height, wind = data[idx]
# 计算新的蔓延范围
new_x = x + spread_speed * math.cos(math.radians(wind)) * time_step
new_y = y + spread_speed * math.sin(math.radians(wind)) * time_step
return new_x, new_y
# 蔓延时间为1800分钟,每5分钟更新一次
for i in range(1, 181):
for j in range(len(data)):
# 如果当前状态为1,则更新周围坐标点的状态
if status[j] == 1:
# 计算蔓延因子
spread_factor = calculate_spread_factor(data[j][3])
# 计算8个方向上的蔓延速度
spread_speeds = [calculate_spread_speed(j-31, spread_factor),
calculate_spread_speed(j-30, spread_factor),
calculate_spread_speed(j-29, spread_factor),
calculate_spread_speed(j-1, spread_factor),
calculate_spread_speed(j+1, spread_factor),
calculate_spread_speed(j+29, spread_factor),
calculate_spread_speed(j+30, spread_factor),
calculate_spread_speed(j+31, spread_factor)]
# 更新周围坐标点的状态
for k in range(8):
new_x, new_y = calculate_spread_range(j, spread_speeds[k], 5)
if new_x >=0 and new_x <= 100 and new_y >=0 and new_y <= 100:
idx = int(new_y) * 100 + int(new_x)
set_status(idx, 2)
# 将状态为2的点更新为1
elif status[j] == 2:
set_status(j, 1)
# 输出结果
for i in range(len(data)):
print(f"坐标({data[i][0]}, {data[i][1]})的状态为{status[i]}")
```
需要注意的是,上述实现中并没有完全按照需求3进行计算蔓延因子,实际应用中需要根据具体情况进行调整。
阅读全文
相关推荐

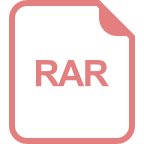
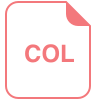
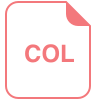






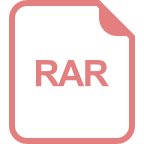
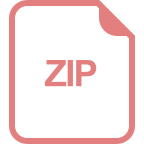
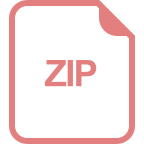
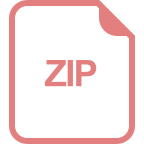
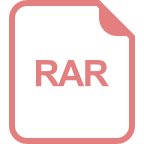
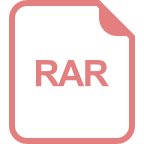