【Python栅格数据可视化技术】:二维到三维的视觉创新之旅
发布时间: 2024-09-12 06:42:05 阅读量: 128 订阅数: 45 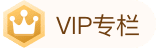
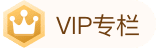
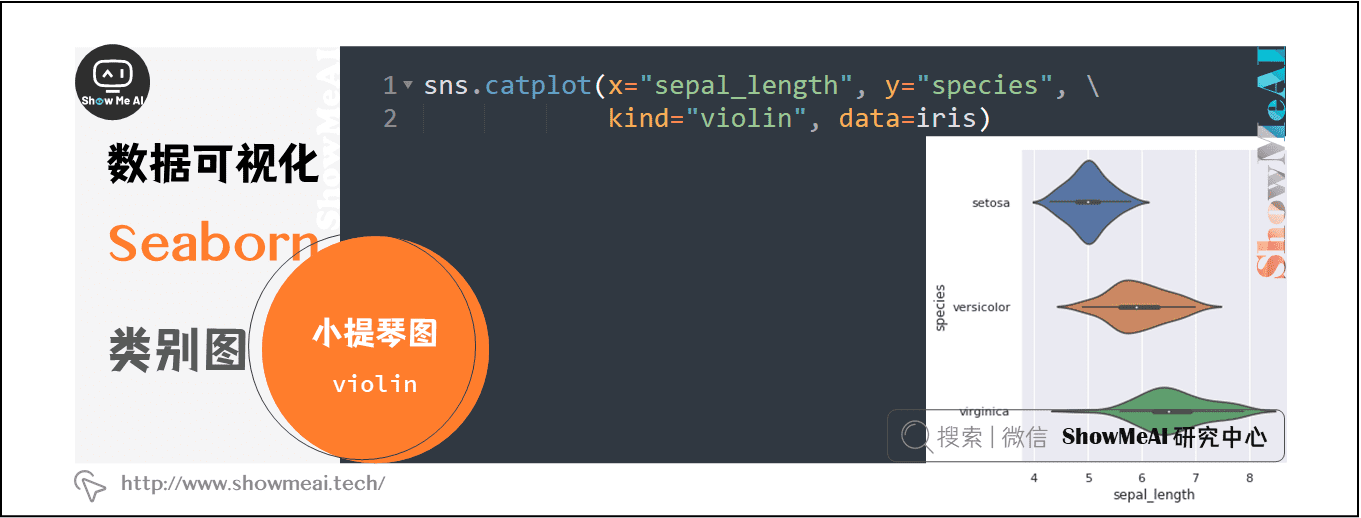
# 1. Python栅格数据可视化概述
## 1.1 数据可视化的重要性
在数据科学的领域,可视化是理解复杂数据集、发现模式、分析趋势和传达信息的关键环节。特别是在处理栅格数据时,将抽象的数字以直观的形式展示出来,能够帮助分析师更有效地进行空间分析和决策制定。
## 1.2 Python在数据可视化中的地位
Python作为一门广泛使用的编程语言,在数据可视化领域同样占据着举足轻重的地位。其强大的第三方库支持,如Matplotlib、Seaborn、Plotly以及专门用于地理空间数据的GeoPandas和PyGMT等,为栅格数据的可视化提供了无限的可能性。
## 1.3 栅格数据的特点与挑战
栅格数据是由网格上的点阵组成,通常用于表示如卫星影像、气象数据等的空间信息。栅格数据的可视化要求处理高维度和大规模数据集,这给数据处理和图形渲染带来了挑战。不过,随着Python库的不断优化和升级,这些挑战正在逐渐被克服。
## 1.4 本章小结
本章作为系列文章的开篇,我们介绍了Python栅格数据可视化的基本概念、重要性以及Python在该领域中的应用和地位。为读者提供了对后续章节所探讨的深入主题的必要背景知识。接下来的章节将带领读者深入学习如何利用Python实现高效且引人注目的数据可视化。
# 2. Python基础数据处理与可视化
## 2.1 Python数据处理入门
### 2.1.1 Python环境搭建与基础语法
Python作为一种广泛使用的高级编程语言,以其易读性和简洁的语法风格而受到开发者的喜爱。在进行数据处理和可视化之前,必须先搭建好Python的运行环境。对于初学者来说,推荐使用Anaconda这一Python发行版本,它包含了Python解释器以及丰富的数据科学相关库,如NumPy、Pandas等。
搭建环境后,需要熟悉Python的基础语法。Python代码是以缩进的方式来组织的,缩进的空格数可以是任意的,但必须一致。Python中的基本数据类型包括:整数、浮点数、字符串、布尔值、列表、元组、字典、集合等。
举例来说,下面的代码段展示了如何创建一个简单的Python程序,该程序会打印出"Hello, World!":
```python
print("Hello, World!")
```
这段代码调用了`print`函数,它是Python的内置函数,用于将信息输出到控制台。在Python中,使用缩进来定义代码块,如上面代码的缩进部分。
### 2.1.2 数据结构与类型在可视化中的应用
数据结构是组织和存储数据的一种方式,它决定了数据如何被存储和访问。在Python中,最常用的数据结构包括列表(List)、元组(Tuple)、字典(Dictionary)和集合(Set)。
列表是一种有序集合,可以随时添加和删除其中的元素。例如,下面的代码创建了一个包含三个元素的列表:
```python
my_list = [1, 'Python', 3.14]
```
元组是另一种有序集合,但与列表不同的是,元组一旦创建便不可修改(不可变)。例如:
```python
my_tuple = (1, 'Python', 3.14)
```
字典是一种键值对集合,通过键来存取对应的值。例如:
```python
my_dict = {'name': 'Alice', 'age': 25}
```
集合是一个无序的不重复元素集。例如:
```python
my_set = {1, 2, 3}
```
在数据可视化中,理解这些基础数据结构的特性非常关键。例如,使用Pandas库可以将这些数据结构转换为Series或DataFrame对象,这些对象为数据处理和可视化提供了更为高级的功能。
## 2.2 基础图表绘制技巧
### 2.2.1 使用Matplotlib创建二维图表
Matplotlib是一个非常流行的Python库,用于创建二维图表。它提供了大量的绘图功能,使得创建高质量图表变得非常简单。
下面的代码段展示如何使用Matplotlib绘制一个简单的折线图:
```python
import matplotlib.pyplot as plt
# 数据准备
x = [0, 1, 2, 3, 4]
y = [0, 10, 20, 30, 40]
# 绘制折线图
plt.plot(x, y, color='blue', marker='o') # 'o' 表示数据点为圆形标记
# 添加标题和轴标签
plt.title("Simple Line Chart")
plt.xlabel("X Axis Label")
plt.ylabel("Y Axis Label")
# 显示图表
plt.show()
```
在这个例子中,我们首先导入了`matplotlib.pyplot`模块,并使用`plot`函数绘制了一条线。参数`color`用于指定线条颜色,`marker`用于指定数据点的样式。
### 2.2.2 Seaborn的高级特性与定制化图表
Seaborn是一个基于Matplotlib的Python绘图库,它提供了更为高级的数据可视化功能。Seaborn让创建复杂图表变得简单,并且通过更高级的默认设置提升了Matplotlib图表的美观度。
下面的代码段展示如何使用Seaborn创建一个分布图:
```python
import seaborn as sns
import matplotlib.pyplot as plt
# 使用Seaborn自带的示例数据集
tips = sns.load_dataset("tips")
# 创建一个直方图和一个核密度估计(KDE)图
sns.histplot(tips['tip'], kde=True, color='red', bins=30)
# 添加图表标题
plt.title("Distribution of Tips")
# 显示图表
plt.show()
```
在这个例子中,我们使用`histplot`函数来绘制数据的直方图,并且添加了核密度估计。Seaborn的图表通常不需要太多的参数设置即可生成美观的图表,这使得它非常适合用于快速生成高质量的可视化成果。
以上是对Python基础数据处理和可视化入门知识的简要介绍。接下来的章节中,我们将深入探讨地理信息系统(GIS)在Python中的应用。
# 3. 二维栅格数据可视化进阶技术
在上一章中,我们学习了基础数据处理和简单的图表绘制技巧,为进一步提升数据的可视化效果,本章节将深入探讨二维栅格数据可视化的进阶技术。我们会介绍热力图、等值线图的实现与应用,同时探究高级可视化库如Plotly和Bokeh在创建动态交互图表和响应式可视化界面中的应用。
## 3.1 复杂数据的二维可视化方法
### 3.1.1 热力图的实现与应用
热力图是表示数据集中变量密度或强度的一种图形表示方法,非常适合展示大规模数据点的分布情况。它们提供了一种直观的方式来理解和分析多变量数据集。
热力图的实现可以借助多种Python库,比如`matplotlib`和`seaborn`。`seaborn`库中的`heatmap`函数能够方便地生成热力图。
```python
import seaborn as sns
import matplotlib.pyplot as plt
# 假设df是一个pandas DataFrame,包含了我们需要可视化的数据
# 其中index和columns对应于数据的行和列标签
plt.figure(figsize=(10, 8)) # 设置图像大小
sns.heatmap(df, annot=True, fmt="f", cmap='viridis') # 创建热力图
plt.show()
```
在上述代码中,`cmap`参数用于指定颜色映射,`annot=True`表示在每个单元格中显示数据值。`fmt="f"`定义了数据值的格式。`plt.figure(figsize=(10, 8))`则是为了优化显示效果,确保图像不会因为数据量大而显得拥挤。
热力图常用于生物学和基因组学领域的基因表达数据可视化,也适用于环境科学、金融等领域的数据展示,帮助研究者快速把握数据的整体分布和某些模式。
### 3.1.2 等值线图的绘制技巧
等值线图是展示二维空间中具有不同等值线的连续值的图形。这种图通常用于气象数据,如温度分布、气压分布等,也广泛应用于地形学,显示等高线、等深线。
使用Python的`matplotlib`库可以绘制等值线图。下面的代码示例演示了如何生成等值线图:
```python
import matplotlib.pyplot as plt
import numpy as np
# 创建一个二维网格,用于计算高度值
x = np.linspace(-3.0, 3.0, 100)
y = np.linspace(-3.0, 3.0, 100)
X, Y = np.meshgrid(x, y)
Z = np.exp(-X**2 - Y**2) - np.exp(-(X-1)**2 - (Y-1)**2)
plt.figure(figsize=(8, 6)) # 设置图像大小
plt.contour(X, Y, Z, 20, cmap='RdGy') # 绘制等值线图
plt.colorbar() # 添加颜色条
plt.show()
```
在这段代码中,`np.meshgrid`用于创建一个二维网格,`X`和`Y`是网格点的横纵坐标,`Z`是每个网格点的高度值。`plt.contour`函数用于绘制等值线,其中`20`指定了等值线的个数。最后,`plt.colorbar()`为等值线图添加了一个颜色条,以便于识别不同颜
0
0
相关推荐
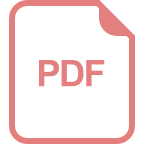
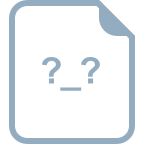
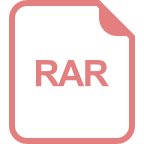





